User Authentication and Session Management in PHP
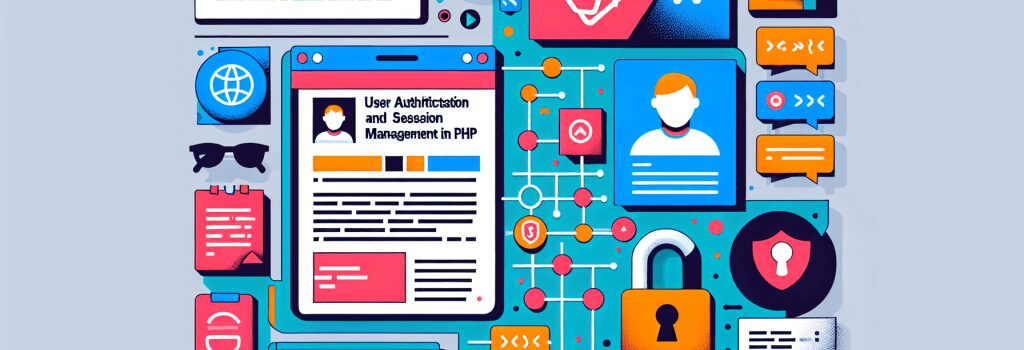
User Authentication and Session Management in PHP
Creating secure and efficient web applications requires a robust system for managing user authentication and session management. PHP, a server-side scripting language, offers powerful features to achieve this, ensuring that only authorized users can access certain parts of your web application. This article provides an in-depth look at implementing user authentication and handling sessions in PHP, aiming to enhance the security and user experience of your dynamic web applications.
Understanding User Authentication
User authentication is a critical component of web security, ensuring that only authenticated users can access specific resources. It involves verifying the identity of users through credentials, typically a username and password. However, authentication can also include other methods like biometric data, one-time passwords (OTP), or security tokens.
Implementing Basic Authentication in PHP
To implement basic user authentication in PHP, follow these steps:
1. Create a Users Database: Store user data, including usernames and hashed passwords, in a database. MySQL is a popular choice for PHP applications.
2. User Registration: Develop a registration form to collect user data. Ensure that passwords are hashed using PHP functions such as ;password_hash()> before storing them in your database.
3. Login Form: Create a login form where users can submit their credentials.
4. Verify Credentials: When a user attempts to log in, fetch the user’s hashed password from the database and compare it using ;password_verify()>. If the verification is successful, the user is authenticated.
Session Management in PHP
Once a user is authenticated, it’s essential to maintain their session to track their interactions with the application. PHP sessions provide a way to preserve certain data across subsequent accesses, which is crucial for maintaining a user’s authenticated state.
Starting a Session
Before any session variables can be used, a session must be started using ;session_start()>. This function should be called at the beginning of your script:
php
<?php
session_start();
?>
Storing and Accessing Session Data
After starting a session, you can store data in the ;$_SESSION> superglobal array. For example, to store a user’s ID:
php
$_SESSION['user_id'] = $user_id;
To access this data later in the session, simply refer back to the ;$_SESSION> array:
php
$user_id = $_SESSION['user_id'];
Managing Session Security
To enhance the security of PHP sessions, consider the following best practices:
– Use HTTPS: Always serve your pages over HTTPS to protect session ID cookies from being intercepted.
– Session Regeneration: Regularly regenerate session IDs using ;session_regenerate_id()> to prevent session fixation attacks.
– Session Timeout: Implement session timeouts to automatically log users out after a period of inactivity.
– Data Validation: Always validate and sanitize user inputs to prevent injections and other attacks.
Logging Out Users
To log out a user, simply unset the session variables and destroy the session using ;session_unset()> and ;session_destroy()>, respectively:
php
<?php
session_start();
session_unset();
session_destroy();
?>
Conclusion
Implementing secure user authentication and session management is vital for protecting your PHP web applications and providing a safe, user-friendly experience. By following the practices outlined in this guide, developers can ensure that their applications are not only secure but also efficient and scalable. Remember, web security is an ongoing process, requiring regular updates and adjustments to tackle new threats as they emerge.