PHP Syntax and Basic Programming Concepts
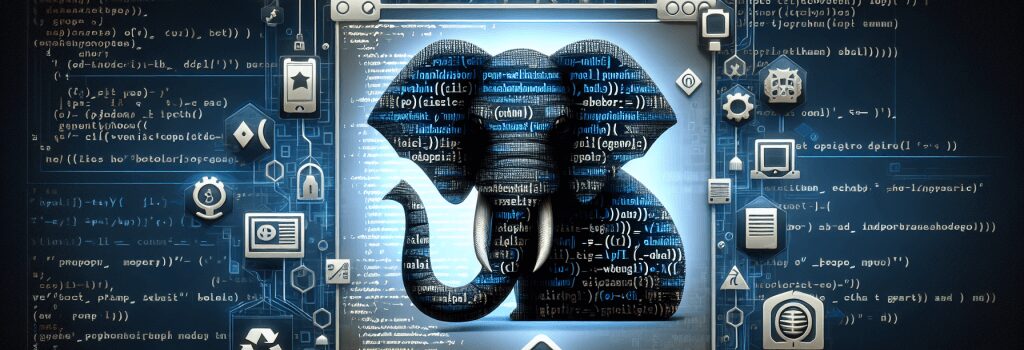
Understanding PHP Syntax and Basic Programming Concepts
When embarking on a journey to become a proficient web developer, mastering the PHP syntax and grasping fundamental programming concepts are foundational steps. PHP (Hypertext Preprocessor) is a server-side scripting language that adds dynamic functionality to websites, enabling tasks such as form processing, content management, and database interaction. In this comprehensive guide, we’ll delve into PHP’s syntax and explore basic programming principles to set you on the right path.
The Basic Structure of PHP
PHP scripts can be easily integrated into HTML. A PHP script begins with ;<?php> and ends with ;?>>. Anything contained within these tags is interpreted as PHP code. For example:
php
<?php
echo "Hello, world!";
?>
This script outputs "Hello, world!" to the web browser, illustrating how PHP can dynamically generate webpage content.
Variables and Data Types
Variables in PHP are declared with a ;$> symbol followed by the variable name. PHP is a loosely typed language, meaning you don’t have to declare the data type of a variable explicitly. It automatically determines the variable’s data type based on its value. The primary data types in PHP include:
– Strings
– Integers
– Floats (floating-point numbers, also known as doubles)
– Booleans
– Arrays
– Objects
– NULL
For example:
php
<?php
$stringVariable = "This is a string";
$integerVariable = 42;
?>
Control Structures: Conditional Statements and Loops
Conditional statements and loops form the core of programming logic in PHP, allowing you to control the flow of execution based on conditions or repeat actions.
Conditional Statements
if, else, and elseif are used to execute different blocks of code based on different conditions.
php
<?php
if ($a > $b) {
echo "a is greater than b";
} elseif ($a == $b) {
echo "a is equal to b";
} else {
echo "a is smaller than b";
}
?>
Loops
PHP supports several types of loops, including for, foreach, while, and do-while, to execute a block of code multiple times.
php
<?php
for ($i = 0; $i < 10; $i++) {
echo $i;
}
?>
Functions
Functions are blocks of code that you can reuse to perform a specific task. In PHP, you can define a function using the ;function> keyword, followed by the function name and a set of parentheses.
php
<?php
function sayHello() {
echo "Hello, world!";
}
sayHello(); // Calls the function
?>
Including Files
PHP enables the inclusion of files to promote code reuse. The ;include> and ;require> statements are used to insert the content of one PHP file into another PHP file before the server executes it.
php
<?php
include 'header.php';
require 'functions.php';
?>
This can significantly streamline your workflow, especially in building complex applications.
Error Handling
Error handling is essential for creating resilient applications. PHP provides several ways to handle errors gracefully, including custom error handlers and exception handling with ;try-catch> blocks.
php
<?php
try {
// Code that may throw an exception
} catch (Exception $e) {
echo 'Caught exception: ', $e->getMessage(), "n";
}
?>
Conclusion
Mastering PHP syntax and understanding its basic programming concepts are crucial for anyone aiming to excel in web development, particularly in backend development. By familiarizing yourself with variables, data types, control structures, functions, file inclusion, and error handling, you are laying a solid foundation for your development skills. As you continue to explore and practice, you’ll find PHP both powerful and versatile, capable of powering everything from small websites to comprehensive web applications like WordPress.
Dive deep, experiment, and remember, proficiency in PHP opens up a vast world of development opportunities. Happy coding!