PHP Arrays: Understanding Indexed and Associative Arrays
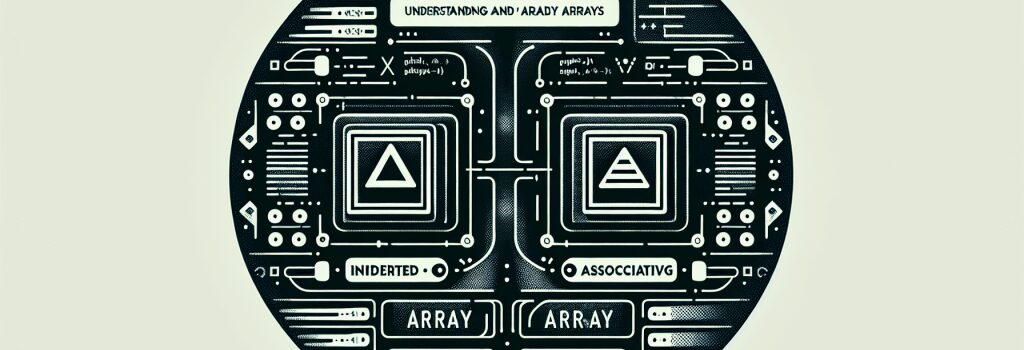
Introduction to PHP Arrays
PHP arrays are a fundamental part of web development, allowing developers to store multiple values within a single variable. Understanding how to effectively work with arrays in PHP can significantly enhance your coding efficiency and capability. This article delves into the basics of PHP arrays, focusing on the two primary types: indexed and associative arrays. We’ll explore how to create, access, and manipulate these arrays to develop dynamic and interactive web applications.
What is an Array in PHP?
An array in PHP is a data structure that allows you to store multiple values in a single variable. It can hold values of any data type, such as integers, strings, or even other arrays. Arrays are incredibly flexible and serve as the backbone for managing collections of data in web development tasks.
Indexed Arrays
Understanding Indexed Arrays
Indexed arrays are the simplest form of arrays in PHP, where each value is automatically assigned a numeric index starting from 0. These are ideal for storing lists of data where the order matters but the specific keys do not.
Creating Indexed Arrays
To create an indexed array in PHP, you can use the ;array()> function or the short array syntax ;[]>. Here is an example:
php
$fruits = array("Apple", "Banana", "Cherry");
// Or using the short array syntax
$fruits = ["Apple", "Banana", "Cherry"];
Accessing Indexed Array Elements
You can access elements of an indexed array by referring to its numeric index. For instance, to access the first element:
php
echo $fruits[0]; // Outputs: Apple
Associative Arrays
Understanding Associative Arrays
Associative arrays are a bit more sophisticated, allowing you to assign your own keys to values. These keys can be strings or integers, making associative arrays ideal for storing data with specific identifiers.
Creating Associative Arrays
Creating an associative array in PHP is similar to creating an indexed array, but you define key-value pairs. Here’s an example:
php
$person = array("firstName" => "John", "lastName" => "Doe");
// Or using the short array syntax
$person = ["firstName" => "John", "lastName" => "Doe"];
Accessing Associative Array Elements
Accessing the elements of an associative array requires you to use the keys you have defined:
php
echo $person["firstName"]; // Outputs: John
Manipulating Arrays
Both indexed and associative arrays can be manipulated in several ways, including adding, removing, and altering elements. PHP provides a plethora of built-in functions for array manipulation, such as ;array_push()>, ;array_pop()>, ;array_shift()>, ;array_unshift()>, and many more, which are crucial for effective array management.
Conclusion
Arrays in PHP, whether indexed or associative, are powerful tools for handling multiple data items within a single variable. Understanding how to create, access, and manipulate these arrays is essential for any aspiring web developer. By mastering PHP arrays, you can efficiently manage complex data structures, making your web applications more dynamic and responsive. With practice, you’ll find arrays to be an indispensable part of your PHP programming toolkit.
Harness the Power of PHP Arrays
Embrace the versatility and functionality of PHP arrays in your web development projects. By understanding the nuanced differences between indexed and associative arrays, you’re well on your way to creating more efficient and readable code. Remember, the key to mastering PHP arrays lies in practice and experimentation, so don’t hesitate to apply what you’ve learned in real-world scenarios.