Operators in PHP: The Building Blocks of Logic
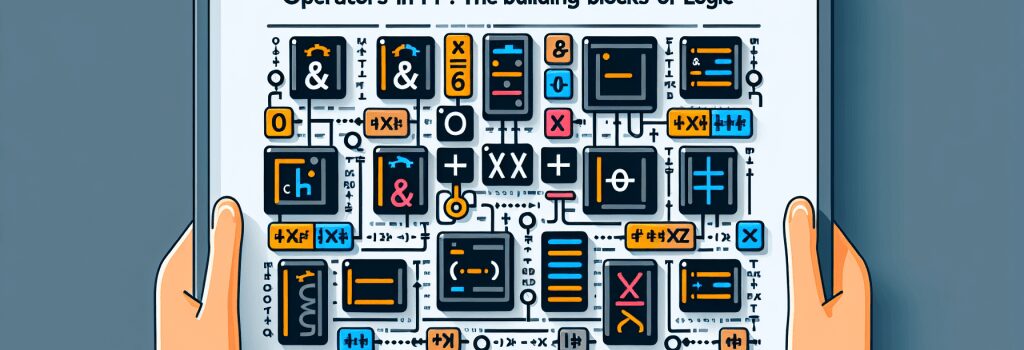
Operators in PHP: The Building Blocks of Logic
When embarking on the journey to become a proficient web developer, understanding the core concepts of PHP is imperative. Among these concepts, operators in PHP hold a pivotal role in constructing the logic that drives dynamic web content. Operators are essentially the building blocks that allow us to perform operations on variables and values, encompassing everything from arithmetic calculations to comparisons and assignment operations. In this article, we delve into the diverse range of operators in PHP, shed light on their categorization, and illustrate their application with examples.
Understanding PHP Operators
PHP operators are symbols that perform operations on operands. An operand can be a value, a variable, or an expression. Based on their functionality, PHP operators are classified into several categories including arithmetic operators, assignment operators, comparison operators, increment/decrement operators, logical operators, string operators, and array operators.
Arithmetic Operators
Arithmetic operators in PHP are used for performing common arithmetic operations. These include:
– ;+> (Addition)
– ;-> (Subtraction)
– ;*> (Multiplication)
– ;/> (Division)
– ;%> (Modulus)
Example:
Assignment Operators
Assignment operators are used to write a value to a variable. The most common assignment operator is ;=>, which assigns the value on the right to the variable on the left.
– ;=> (Basic assignment)
– ;+=> (Add and assign)
– ;-=> (Subtract and assign)
Example:
Comparison Operators
Comparison operators allow you to compare two values and return a Boolean value (;true> or ;false>) based on the comparison.
– ;==> (Equal)
– ;===> (Identical)
– ;!=> (Not equal)
– ;>> (Greater than)
Example:
Increment/Decrement Operators
These operators are used to increment or decrement a variable’s value.
– ;++> (Increment)
– ;–> (Decrement)
Example:
Logical Operators
Logical operators are used to combine conditional statements.
– ;and> (&&)
– ;or> (||)
– ;!> (Not)
Example:
String Operators
String operators are specifically designed for manipulating strings.
– ;.> (Concatenation)
– ;.=> (Concatenate and assign)
Example:
Array Operators
Array operators allow you to compare and operate on arrays.
– ;+> (Union)
– ;==> (Equality)
– ;===> (Identity)
Example:
In Conclusion
Operators in PHP are fundamental in the development of web applications. They enable developers to perform arithmetic operations, manipulate string and array data, make comparisons, and logically control the flow of code. By understanding and effectively utilizing these operators, you can significantly enhance your programming logic and contribute to more efficient and powerful web development projects. As you continue your journey in becoming a skilled web developer, keep experimenting with these operators in different scenarios to deepen your understanding and proficiency.