Mastering String Variables in PHP
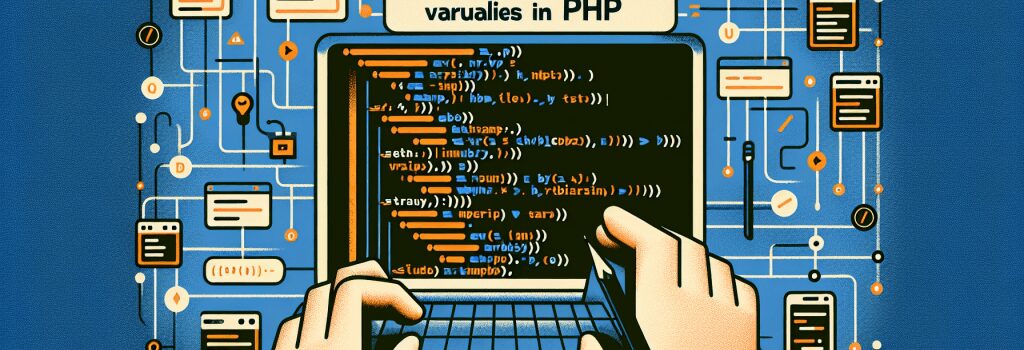
Mastering String Variables in PHP
In the realm of web development, PHP stands as a cornerstone technology, empowering developers to create dynamic and interactive websites. Among the essential concepts in PHP that developers must understand, string variables hold a significant place. This guide is meticulously crafted to help you master string variables in PHP, enhancing your backend development skills.
Understanding String Variables in PHP
In PHP, a string is a sequence of characters, where each character is the same as a byte. This means that PHP supports a 256-character set, and hence, it can work with a wide array of languages and symbols. String variables are used to store text, and they can include letters, numbers, spaces, and symbols.
Declaring String Variables
String variables in PHP can be declared and initialized in two ways:
1. Single Quoted Strings: Ideal for plain text, single quoted strings will literally output the text they contain, interpreting escape sequences like ;’> (single quote) and ;> (backslash) only.
2. Double Quoted Strings: Double quoted strings interpret a variety of escape sequences and variable names within the string.
Concatenation of Strings
Combining two or more strings in PHP is known as concatenation, achieved using the ;.> operator. This is a pivotal technique in dynamic content generation.
Common String Functions
PHP offers a plethora of built-in functions to manipulate strings, making it a powerful tool for developers. Here are some commonly used string functions:
– ;strlen()>: Returns the length of a string.
– ;str_word_count()>: Counts the number of words in a string.
– ;str_replace()>: Replaces some characters in a string with some other characters.
– ;strpos()>: Finds the position of the first occurrence of a substring in a string.
Handling Multibyte String Functions
In an era of global web applications, dealing with multibyte characters, such as characters from the Japanese or Chinese alphabets, is common. PHP’s Multibyte String extension provides functions that properly handle multibyte characters, ensuring your applications are truly international.
In Conclusion
Mastering string variables in PHP is a fundamental step toward becoming proficient in backend web development. Through understanding, declaring, manipulating, and handling strings, developers can create dynamic and interactive web applications. As you dive deeper into PHP, remember that strings are not just sequences of characters but are the building blocks of content on the web.
Embarking on this journey towards mastering PHP, keep experimenting with string functions and pay attention to how strings interact within the broader context of your web applications. With practice and dedication, you’ll soon leverage the full power of strings in PHP to create compelling and user-friendly web experiences.