Mastering Multidimensional Arrays in PHP
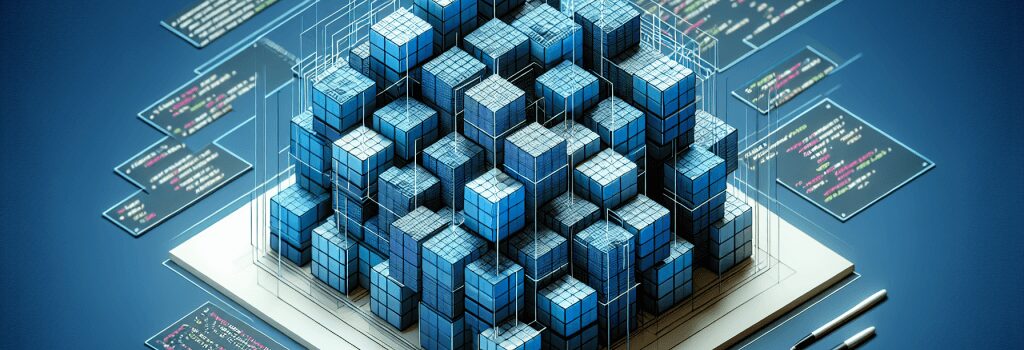
In the quest to become an accomplished web developer, mastering the intricacies of PHP, especially when it comes to data management, is crucial. Among the most powerful tools in PHP’s arsenal are arrays, particularly multidimensional arrays. These structures allow you to manage complex data sets effectively, making your backend development tasks significantly more manageable.
Understanding Multidimensional Arrays in PHP
At its core, a multidimensional array in PHP is simply an array whose elements are arrays themselves. This structure can represent various types of data, such as tables or matrices, in a compact and accessible form. Understanding how to create, access, and manipulate these arrays is essential for any developer looking to implement sophisticated data handling capabilities in their applications.
Creating Multidimensional Arrays
Creating a multidimensional array in PHP is straightforward. You define an array, with each of its elements being another array containing its own set of elements. For example, to represent a simple table of user data, you might set up an array like this:
In this structure, each sub-array represents a user, containing their name, age, and email address. This is a basic two-dimensional array, but the concept extends to even more dimensions, depending on the complexity of the data you’re managing.
Accessing Data Within Multidimensional Arrays
Accessing the data stored in multidimensional arrays is as simple as chaining array keys. For example, to access Jane Smith’s age in the ;$users> array mentioned above, you would use:
This notation can be extended to access deeper levels in more complex arrays, allowing you to navigate through layers of data efficiently.
Manipulating Multidimensional Arrays
PHP offers a wide range of functions to manipulate arrays, many of which can be applied to multidimensional arrays. Functions like ;array_map()>, ;array_filter()>, and ;array_reduce()> can be particularly useful when working with these structures. However, applying these functions to multidimensional arrays often requires the use of callbacks to achieve the desired operation on every element or specific layers of the array.
Best Practices for Working with Multidimensional Arrays
When dealing with multidimensional arrays, keeping your code clean and understandable is key. Here are some practices to help you manage these structures effectively:
– Use descriptive keys: Associative arrays allow you to use string keys, which can make your data significantly easier to understand and access correctly.
– Keep dimensions manageable: While PHP supports arrays with multiple levels of depth, deeper arrays can become difficult to manage and understand. Try to simplify your data structure where possible.
– Leverage built-in functions: PHP’s array functions are powerful. Explore them fully to simplify your array manipulation code.
– Document your data structures: Especially with complex multidimensional arrays, documenting the structure and purpose of each level can be invaluable for both you and other developers.
Conclusion
Multidimensional arrays in PHP are a fundamental concept that every aspiring web developer should master. They form the backbone of complex data manipulation and handling in backend development. By understanding how to create, access, and manipulate these arrays, developers can handle a vast array of data management tasks more efficiently. Following best practices and utilizing PHP’s array functions can greatly simplify working with these complex structures, making your code more readable and maintainable.