Manipulating Data with PHP: A Guide to Using Operators
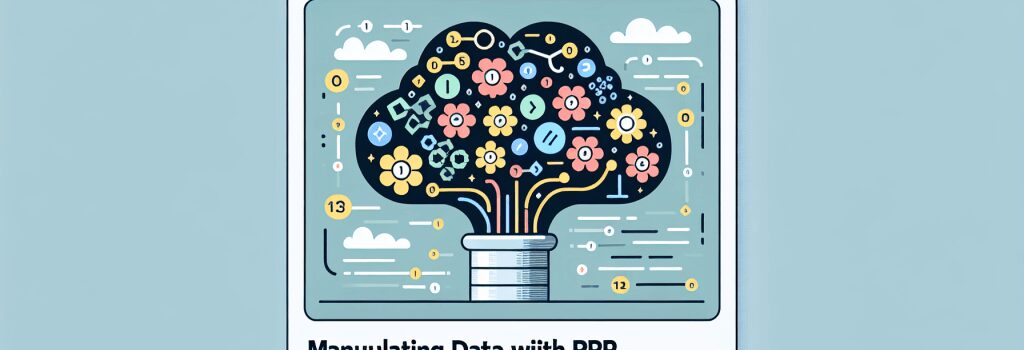
Understanding PHP Operators: The Key to Effective Data Manipulation
PHP, a pivotal server-side scripting language used in web development, enables the creation of dynamic and interactive web pages. At the core of PHP programming are operators— tools that allow you to perform operations on variables and values. Grasping the concept of PHP operators is essential for anyone looking to master backend development. This guide dives deep into the different types of operators in PHP and demonstrates how to harness their power to manipulate data efficiently.
The Essence of PHP Operators
Operators are symbols that instruct PHP to perform a specific operation or computation. They play a crucial role in processing data, making decisions, and controlling the flow of a PHP application. Understanding how to use operators effectively will enable you to manipulate data in a variety of ways, such as performing mathematical calculations, comparing values, and assigning data to variables.
Types of Operators in PHP
PHP provides a wide range of operators, each serving distinct purposes. These operators are broadly classified into the following categories:
Arithmetic Operators
Arithmetic operators are used for basic mathematical operations. They include:
– Addition (;+>): Adds two values together.
– Subtraction (;->): Subtracts one value from another.
– Multiplication (;>): Multiplies two values.
– Division (;/>): Divides one value by another.
– Modulus (;%>): Finds the remainder of a division.
– Exponentiation (;<strong></strong>>): Raises one value to the power of another.
Assignment Operators
Assignment operators are used to assign values to variables. The basic assignment operator is ;=>, which assigns the value on the right to the variable on the left. There are also compound assignment operators that combine an arithmetic operator with the assignment operator, such as ;+=>, ;-=>, ;=>, ;/=>, and ;%=>.
Comparison Operators
Comparison operators allow you to compare two values. The result of a comparison is a boolean value (;true> or ;false>). Common comparison operators include:
– Equal (;==>): True if the two values are equal.
– Identical (;===>): True if the two values are equal and of the same type.
– Not Equal (;!=>): True if the two values are not equal.
– Not Identical (;!==>): True if the two values are not equal or not of the same type.
– Greater Than (;>>): True if the value on the left is greater than the value on the right.
– Less Than (;<>): True if the value on the left is less than the value on the right.
Logical Operators
Logical operators are used to combine conditional statements. They include:
– And (;&&>): True if both conditions are true.
– Or (;||>): True if at least one of the conditions is true.
– Not (;!>): True if the condition is not true.
Manipulating Data with Operators
PHP operators are invaluable tools for manipulating data. For instance, you can use arithmetic operators to perform calculations on user input or variables. Assignment operators can be used to update the value stored in a variable. Comparison operators are crucial for decision-making processes, such as validating user inputs or determining the flow of a script based on certain conditions. Logical operators enable you to construct complex conditional statements, enhancing the decision-making capability of your PHP scripts.
Best Practices for Using PHP Operators
When using operators in PHP, it’s important to follow some best practices:
– Understand Operator Precedence: Operator precedence defines the order in which operations are performed. Knowing the precedence ensures that expressions are evaluated in the order you intend.
– Use Parentheses for Clarity: Parentheses can be used to explicitly specify the order of operations. This not only ensures correct evaluation but also improves the readability of the code.
– Be Mindful of Data Types: When performing operations, especially comparisons, be aware of the data types of the values involved to avoid unexpected results.
Mastering the use of operators is a fundamental step towards becoming proficient in PHP and, ultimately, a skilled web developer. Through practical application and experimentation, you’ll become comfortable with these powerful tools, enabling you to manipulate data effectively and build dynamic, data-driven web applications.