How to Work with File I/O in PHP
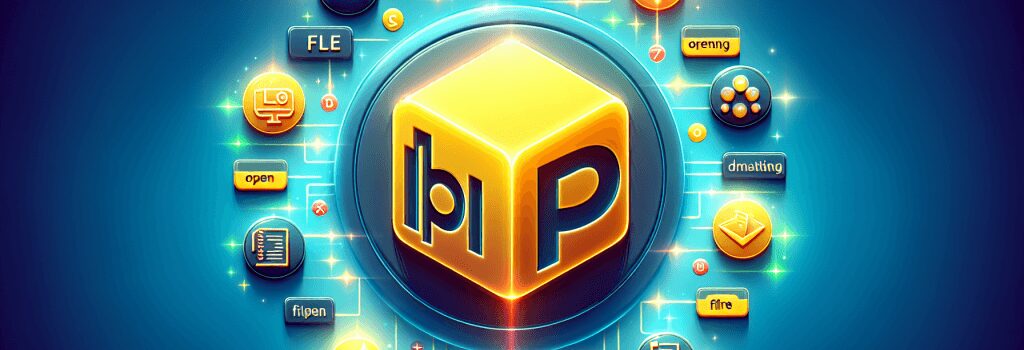
Introduction to File I/O in PHP
Welcome to another fun chapter of learning PHP. Today we’ll be diving into a front seat viewing of the magical world of File Input/Output (I/O) operations in PHP. Now, don’t get lost in the fancy name – it’s much easier than it sounds. Imagine you’re at a fast food drive-thru, you place your order (input), and then you receive your bundle of joy, the oversized burger (output). That’s a simple example of I/O operations in life.In PHP, File I/O operations are about reading data from files (input) or writing data to files (output). You might be asking ‘why would I need to do that?’ Well, hang tight because we’re just getting started.
Why Do we Need File I/O in PHP?
Think about a scenario where you have a high score system for a game on your website. You would need to save the data, right? Now imagine the horror if you couldn’t save that world-record-breaking score of yours, tragic right? That’s where PHP File I/O operations come in to save the day (and your high score)!Getting Started with File I/O in PHP
Let’s dive right in. Like Nemo in the great blue, we’re about to explore some thrilling adventures with PHP.Opening a File
Opening a file is like greeting it. You know, a friendly ‘Hi, file!’, before we start messing with it. You do this with the ;fopen()> function. It’s as eager to get started as you are.Code Snippet:
$file = fopen(“your_file.txt”, “r”);
‘R’ stands for reading mode. This means we just opened a file for reading. There are other modes as well, like ‘w’ for writing, ‘a’ for append and more.
Reading a File
After you’ve greeted your file, it’s time to find out what’s inside. Like the inside of a taco, it’s like a fun surprise! PHP’s ;fread()> functions lets you do just that.Code Snippet:
$content = fread($file,filesize(“your_file.txt”));
This reads the entire file. Have a big file? PHP doesn’t care! It’ll read the whole file faster than you can say ‘supercalifragilisticexpialidocious’.
Writing a File
And now we come to the main event! So, you’ve got some data and you just can’t wait to save it to a file. Well, the ;fwrite()> function’s got your back.Code Snippet:
$file = fopen(“your_file.txt”, “w”);
fwrite($file, “Hello, world!”);
This will open your file in write mode (wiping out anything that was in it previously) and write ‘Hello, world!’ to it.
Conclusion
Boom! You’ve now learned about magical PHP world of File I/O. You can now open, read, and write files. Remember, with great power comes great responsibility-understand what each function does before using it. Now go on, start working with files, decide their fate, become the File Master, the PHP File Pupil!Remember, practice makes perfect, so it wouldn’t hurt to write a little code here and there to familiarize yourself with these concepts. There’s nothing like a good old coding session to cement new knowledge.
Let’s meet again in the next session as we continue to explore the intriguing world of PHP. Till then, keep your coding skills sharp!
FAQ
What is file I/O in PHP?
File I/O stands for Input/Output operations on files, which allow PHP to read from and write to files on the server’s filesystem. It is essential for handling data persistence and storage.
How can I open a file for reading in PHP?
You can use the `fopen()` function to open a file for reading in PHP. The syntax is `fopen(“filename.txt”, “r”)`, where “filename.txt” is the name of the file you want to read.
How do I read the contents of a file in PHP?
You can use functions like `fread()` or `fgets()` to read the contents of a file in PHP. These functions allow you to read the file line by line or a specific number of bytes at a time.
Can I write to a file using PHP?
Yes, you can write to a file using PHP by opening the file in write or append mode. You can use functions like `fwrite()` to write data to a file or `file_put_contents()` to write data in a more concise way.
How do I check if a file exists in PHP?
You can use the `file_exists()` function to check if a file exists in PHP. This function returns true if the file exists and false if it doesn’t.
Is it possible to create a new file in PHP?
Yes, you can create a new file in PHP using the `fopen()` function with the “w” mode to open the file for writing. If the file does not already exist, PHP will create it for you.
How can I close a file after I am done working with it in PHP?
You can use the `fclose()` function to close a file after you are done working with it in PHP. It is good practice to close the file to free up system resources and prevent any data corruption.
What should I do if I encounter errors while working with file I/O in PHP?
You should always handle errors gracefully by using functions like `feof()` to check for the end of the file, `feof()` to clear file pointers, and `fclose()` to close the file properly. Additionally, you can use error handling techniques like try-catch blocks to manage exceptions.
Can I read and write CSV files in PHP?
Yes, you can read and write CSV files in PHP using functions like `fgetcsv()` to read a line from a CSV file and `fputcsv()` to write an array to a CSV file. CSV files are a common format for storing tabular data, and PHP provides built-in support for them.
How can I handle large files in PHP without running out of memory?
To handle large files in PHP without running out of memory, you can use functions like `fopen()`, `fread()`, and `fwrite()` to read and write files in smaller chunks. This way, you can process the file gradually instead of loading the entire content into memory at once.