Exploring PHP Control Structures: Break and Continue in Loops
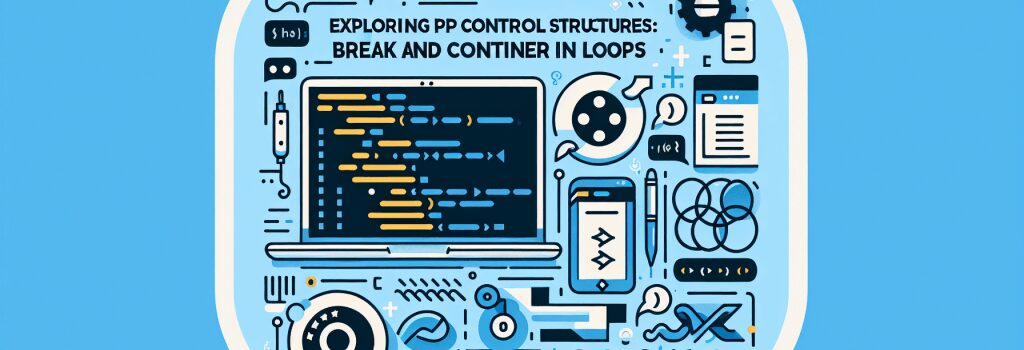
Introduction to PHP Control Structures
In the journey of becoming a proficient web developer, understanding the core principles of backend development is vital. PHP, a server-side scripting language, stands out for its ease of use and wide adoption in web development. One fundamental aspect of mastering PHP involves getting comfortable with its control structures, especially when dealing with loops. This article dives deep into two critical control structures: ;break> and ;continue>, which are essential tools for managing the flow of loops in PHP.
Understanding Loops in PHP
Before exploring the ;break> and ;continue> statements, it’s essential to grasp the basics of loops in PHP. Loops are used to execute the same block of code repeatedly as long as a specified condition is true. PHP supports several types of loops:
– While Loops: Execute the code block as long as the specified condition is true.
– Do-While Loops: Execute the code block once, and then continue executing it as long as the specified condition is true.
– For Loops: Ideal for when you know in advance how many times you want to execute a statement.
– Foreach Loops: Used to loop through arrays and objects.
PHP Break Statement
When to Use the Break Statement
The ;break> statement is used to terminate the execution of a loop prematurely. It’s particularly useful when you need to stop the loop based on a specific condition other than the loop’s original terminating condition.
<h4>Example UsageIn the example above, the loop will terminate when ;$i> equals 5, even though the original condition allows it to run until ;$i> is less than 10. This demonstrates how the ;break> statement can provide additional control over your loops’ execution.
PHP Continue Statement
When to Use the Continue Statement
Contrary to ;break>, the ;continue> statement is used to skip the rest of the loop iteration and proceed with the next cycle of the loop. It’s incredibly useful when you encounter an iteration that, for some reason, shouldn’t fully execute but doesn’t justify terminating the entire loop.
<h4>Example UsageIn this scenario, when ;$i> equals 5, the ;continue> statement causes the loop to skip the rest of that particular iteration, avoiding the ;echo> command for ;$i> value of 5, and proceeds with the next iteration of the loop.
Best Practices for Using Break and Continue
While both ;break> and ;continue> statements are powerful tools for controlling the execution flow of loops, it’s essential to use them judiciously. Overuse or incorrect application can lead to code that’s difficult to read and debug. Here are a few best practices:
– Use ;break> and ;continue> only when necessary. Always consider if the same result could be achieved with a loop’s condition itself.
– Avoid deeply nested loops with multiple ;break> or ;continue> statements, as they can make your code less intuitive.
– Document your use of ;break> and ;continue> with comments to ensure that the intention behind their use is clear to others (or to yourself when you return to the code in the future).
Conclusion
Mastering the use of ;break> and ;continue> statements in PHP enhances your ability to control the execution flow within loops, making your backend development tasks more efficient and your code more readable. Remember, the power of these control structures lies in their judicious use, complementing the logical conditions of your loops for optimal code performance and maintainability. As you continue on your path to becoming a well-rounded web developer, incorporating these practices into your PHP coding will undoubtedly set you apart.
By understanding and applying these advanced control structures, you equip yourself with valuable tools that refine your programming logic and contribute to your evolution as a backend developer, further paving your way in the ever-evolving landscape of web development.