Efficient String Handling and Manipulation in PHP
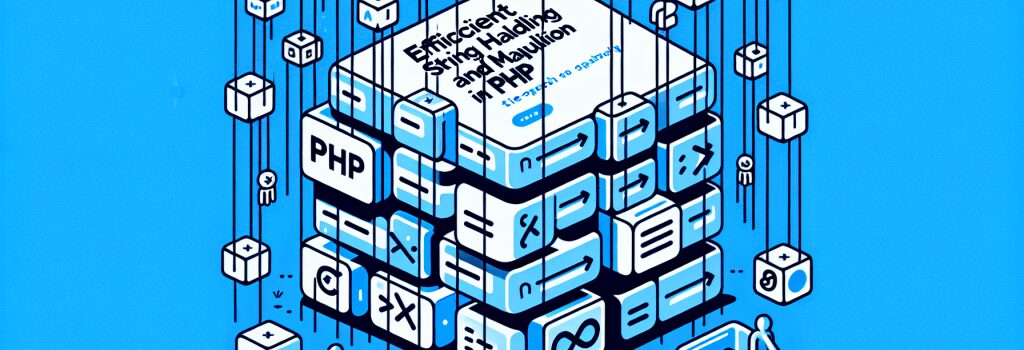
Introduction to String Handling in PHP
String handling is a crucial aspect of web development, particularly in backend development where data manipulation, processing, and presentation play a key role. PHP, a widely-used server-side scripting language, offers a rich set of built-in functions for efficient string handling and manipulation. Mastering these functions can significantly enhance the functionality and performance of your web applications. This article explores the essential techniques for working with strings in PHP, including common functions, best practices, and practical examples.
Understanding PHP Strings
In PHP, a string is a sequence of characters where each character is the same as a byte. This means that PHP supports a 256-character set, which allows for a wide range of characters and symbols to be used within strings. There are four ways to represent strings in PHP: single-quoted, double-quoted, heredoc syntax, and nowdoc syntax, each with its specific use cases and features.
Key PHP String Functions
PHP provides a plethora of functions for string manipulation, making it easy to perform operations such as searching, replacing, splitting, and formatting strings. Here are some of the most commonly used PHP string functions:
String Searching
– ;strpos()>: Finds the position of the first occurrence of a substring in a string.
– ;stripos()>: Case-insensitive version of ;strpos()>.
– ;strstr()>: Finds the first occurrence of a substring in a string and returns the rest of the string.
String Replacement
– ;str_replace()>: Replaces all occurrences of a search string with a replacement string.
– ;str_ireplace()>: Case-insensitive version of ;str_replace()>.
String Formatting
– ;sprintf()>: Formats a string according to specified formatting rules.
– ;number_format()>: Formats a number with grouped thousands.
String Splitting and Joining
– ;explode()>: Splits a string by a specified delimiter.
– ;implode()>: Joins array elements with a string.
Best Practices for String Manipulation
1. Use Single-Quotes for Simple Strings: Single-quoted strings are processed slightly faster than double-quoted strings, as they do not interpret escape sequences or variable values.
2. Sanitize User Input: Always sanitize user-provided data to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS). Functions like ;htmlspecialchars()> and ;strip_tags()> can be helpful.
3. Utilize Multibyte String Functions for UTF-8 Support: Standard string functions do not properly handle multibyte characters (like those in UTF-8). Use the ;mb_*> functions (e.g., ;mb_strlen()>, ;mb_substr()>) for accurate results with multibyte characters.
4. Optimize String Functions Usage: When working with large datasets or within loops, optimize your code by minimizing the use of intensive string functions and considering alternative algorithms or PHP extensions like PCRE (Perl Compatible Regular Expressions) for complex pattern matching and manipulation.
Practical Example: Email Validation and Formatting
One common task in web development is email validation and formatting. Here’s a simple example using PHP string functions:
This example demonstrates using ;strtolower()> for case normalization and ;filter_var()> with the ;FILTER_VALIDATE_EMAIL> flag for validation.
Conclusion
Efficient string handling and manipulation in PHP are fundamental to developing dynamic, data-driven web applications. By mastering PHP’s built-in string functions and adhering to best practices, developers can ensure their applications process and manage data effectively, leading to improved performance and user experience. With the techniques discussed in this article, you are now equipped to tackle string-related tasks in your PHP development projects with confidence.