Debugging and Testing PHP Code: Ensuring Reliability and Performance
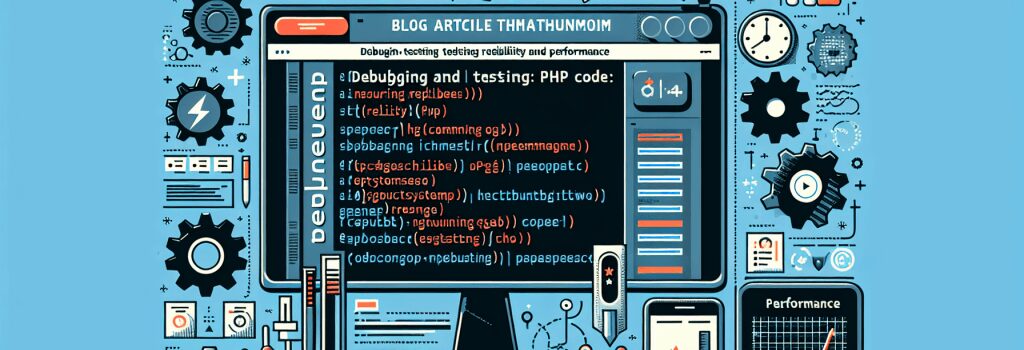
Debugging and testing your PHP code is a critical step in the web development process, ensuring the reliability and performance of your applications. With the right strategies and tools, you can identify bugs early, optimize your code, and enhance the overall user experience. This article covers essential techniques and practices for effectively debugging and testing PHP code.
Importance of Debugging and Testing
Before diving into specific techniques, it’s important to understand why debugging and testing are indispensable parts of PHP development:
– Detecting Errors Early: Identifying and fixing errors early in the development cycle saves time and resources.
– Ensuring Code Quality: Regular testing guarantees that your code meets the required standards and performs as expected.
– Improving Performance: Debugging helps optimize code, improving the efficiency and speed of your PHP applications.
– Enhancing Security: Testing uncovers vulnerabilities, enhancing the security of your applications.
Debugging PHP Code
Debugging involves identifying and removing errors or bugs from your code. PHP offers several tools and techniques to facilitate this process:
Error Reporting
PHP has built-in error reporting functions that can be very helpful in debugging. You can enable error reporting by adding the following lines at the beginning of your script:
php
ini_set('display_errors', 1);
error_reporting(E_ALL);
This configuration makes all types of errors visible during the development stage.
Use of Xdebug
Xdebug is a powerful debugging extension for PHP. It provides a wealth of information to help you understand what’s going on in your code. Features include stack traces, profiling, and code coverage analysis. Installing and configuring Xdebug can substantially reduce the time spent on debugging.
Debugging PHP with IDE
Integrated Development Environments (IDEs) like PhpStorm and Visual Studio Code offer built-in debugging capabilities. These tools allow you to set breakpoints, inspect variable values, and step through your code line by line.
Testing PHP Code
Testing ensures your code works as expected under various conditions. The two primary types of testing in PHP development are:
Unit Testing
Unit testing involves testing individual components or functions of your PHP application. PHPUnit is a popular testing framework designed for PHP. Writing tests with PHPUnit ensures that your functions perform correctly under different scenarios.
Integration and Functional Testing
Integration testing checks how different parts of your application work together, while functional testing evaluates the application from the end-user’s perspective. Tools like Behat and Codeception are great for these types of testing, allowing you to simulate user actions and test the application as a whole.
Best Practices for Debugging and Testing PHP Code
– Develop a Consistent Testing Routine: Make testing a regular part of your development process to catch issues early.
– Write Testable Code: Design your code in a way that makes it easy to test. This often involves using modular, decoupled components.
– Leverage Continuous Integration (CI): CI tools can automatically run your tests and provide feedback with each commit, helping to maintain code quality.
– Learn from Mistakes: Use bugs and errors as learning opportunities to improve your coding practices.
In conclusion, debugging and testing are vital for developing high-quality PHP applications. By implementing the techniques and practices outlined above, you’ll enhance the robustness, performance, and security of your web applications. Remember, a successful web developer constantly refines their debugging and testing skills as part of their ongoing professional development.