Custom Functions in PHP: Creation and Practical Applications
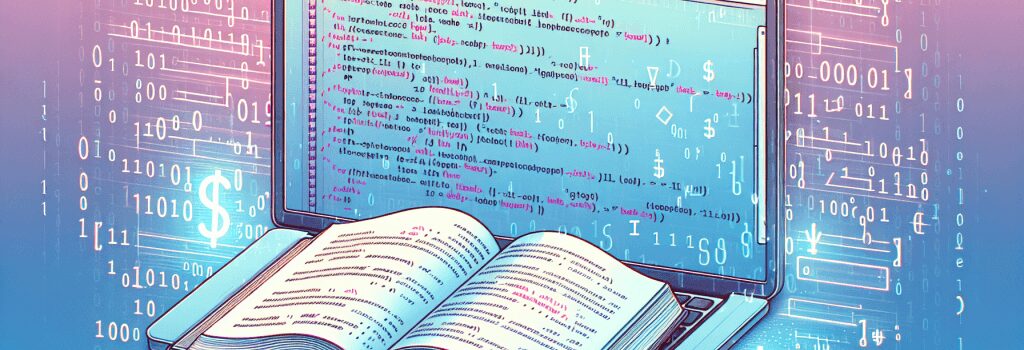
Introduction
Custom Functions in PHP – What’s the Fuss?
Before we dive into the wonderful world of writing custom functions, let’s think about PHP. PHP is like the language of web development. It’s also one of the most misunderstood languages.I mean, imagine if everyone thought English was only good for ordering fast food! That’s the kind of rep PHP often gets. But here’s the thing: it’s not the language, it’s how we use it. The same applies to PHP.
So What’s Up with Custom Functions?
Now if PHP is the language, think of functions as our sentences – succinct, effective and reusable. Yes, reusable! You don’t have to rewrite the same set of codes again and again. Sounds pretty neat, right? Now, sit tight because we’re going to dive right into the how’s of creating those PHP custom functions and open up an exciting new chapter in your web development journey.Playing with PHP: Custom Functions 101
-Just like how burgers need buns and patties, a custom function needs a name (preferably something that tells us what it does) and some brackets with potential variables inside.Imagine a simple PHP function as a short-order chef flipping burgers. You want a burger, you ask the chef, and voila, there is your burger. How does the chef know to make a burger? Because you gave the order – or in coding terms, called the function!
Consider this basic function:
<pre><code>
function flipBurger() {
echo "Here's your yummy hamburger!";
}
To use this function or to "call" it when an order comes, the following line of code is used:
<pre><code>
flipBurger();
You just created and used your very first custom function in PHP!
Practical Applications of Custom Functions in PHP
Writing and using custom functions can make your code cleaner, tighter, and much more manageable. Plus, who doesn’t love recycling? It’s good for the planet and for the code!A fair warning: once you start using custom functions, there’s no turning back – it’s that effective!
Putting PHP Custom Functions into Play
Here’s an example to move you from amateur PHP speaker to a seasoned chatterbox.For instance, let’s think about a task like calculating the area of a rectangle. It’s not complicated math, is it? Length times width, and BOOM! With a custom function in PHP, it would look something like this :
<pre><code>
function rectangleArea($length, $width) {
$area = $length * $width;
echo "The area of your rectangle is $area!";
}
rectangleArea(5,4);
There’s a lot more PHP power where this came from. Your web development journey has only begun!
Final Words
Goodbye pen and paper, hello keyboard! With PHP custom functions, get ready to save time like a pro and avoid code repetition. Custom functions are here to make your coding life easier, cleaner, and faster. Just remember, practice makes perfect when it comes to custom functions. So start coding, start practicing!Congratulations, you’ve now graduated into the wondrous world of writing custom functions in PHP. Happy coding!
Just remember, a semi-colon (;) is the full stop of coding. You forget it, PHP will get grumpy and your code won’t run!
FAQ
How can I create custom functions in PHP?
To create a custom function in PHP, you can use the `function` keyword followed by the name of your function, parameters enclosed in parentheses, and the code block containing the function’s logic enclosed in curly braces.
How do I call a custom function in PHP?
To call a custom function in PHP, simply use the function name followed by parentheses and any required parameters inside the parentheses.
Can I pass arguments to a custom function in PHP?
Yes, you can pass arguments to custom functions in PHP by defining parameters within the function definition. These parameters can then be utilized within the function’s code block.
What is the purpose of creating custom functions in PHP?
Custom functions in PHP allow you to encapsulate reusable blocks of code, improving code organization, readability, and maintainability within your PHP applications.
How do I return a value from a custom function in PHP?
To return a value from a custom function in PHP, you can use the `return` keyword followed by the value that you want to return. This value can then be used wherever the function is called.
Can custom functions in PHP have default parameters?
Yes, you can define default parameter values for custom functions in PHP. When a default value is specified for a parameter and no value is passed when the function is called, the default value is used.
How can I include custom functions from another file in PHP?
You can include custom functions from another PHP file using the `include` or `require` statements, specifying the file path to the PHP file containing the custom functions.
Can I pass functions as arguments to other functions in PHP?
Yes, in PHP, you can pass functions as arguments to other functions. This allows for greater flexibility and functionality in your code, commonly seen in higher-order functions and callback functions.
How do I debug custom functions in PHP?
To debug custom functions in PHP, you can use tools like `var_dump()`, `print_r()`, or `echo` statements to output variable values and troubleshoot issues within your functions.
Are there any naming conventions to follow when creating custom functions in PHP?
It’s recommended to use descriptive and readable names for custom functions in PHP, following naming conventions like camelCase or snake_case to improve code clarity and maintainability.