Converting Between Arrays and Strings in PHP
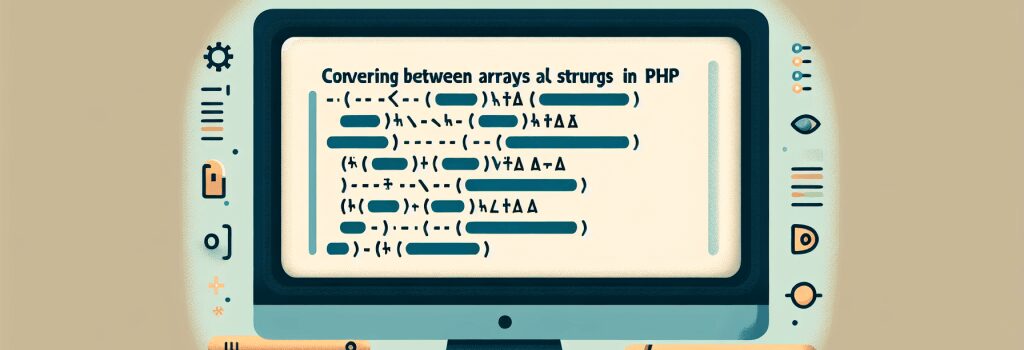
Understanding Arrays and Strings in PHP
PHP, a server-side scripting language, is a cornerstone for many developers aiming to deliver dynamic content on the web. Whether you are a beginner or brushing up your skills, mastering arrays and strings is fundamental. This piece delves into efficiently converting between arrays and strings in PHP— a skill that enhances data manipulation and contributes to cleaner, more effective code.
What Are Arrays and Strings?
Arrays in PHP are complex data types that allow you to store multiple values in a single variable. They come in three types: indexed arrays, associative arrays, and multidimensional arrays. Arrays are versatile, making them an essential tool for any PHP developer.
Strings, on the other hand, are sequences of characters, like words or sentences. In PHP, strings are used to manage text, whether it’s echoing HTML to the browser or manipulating data.
Converting Arrays to Strings
The ;implode()> Function
To convert an array into a string, PHP offers the ;implode()> function. It joins array elements with a string. The syntax is simple:
– The ;separator> is the string to join the array elements with.
– The ;array> is, of course, the array you wish to convert.
Example:
Converting Strings to Arrays
The ;explode()> Function
Converting a string back to an array is just as straightforward with the ;explode()> function. This function splits a string by a specified delimiter, turning it into an array.
– The ;separator> defines where the string should be split.
– The ;string> is the input that you want to split into an array.
Example:
Advanced Techniques
Working with JSON: PHP has built-in functions for encoding and decoding JSON. This is extremely useful when dealing with APIs or wanting to convert multidimensional arrays to strings and vice versa.
– To convert an array to a JSON string, use ;json_encode()>.
– To convert a JSON string to an array, use ;json_decode()>.
Custom Delimiters: When using ;implode()> and ;explode()>, choosing an appropriate delimiter is crucial. For complex data, consider using a delimiter that does not appear in the data to avoid errors in conversion.
Conclusion
Mastering the conversion between arrays and strings in PHP is a valuable skill that enhances your ability to manipulate and work with data. By learning to use ;implode()>, ;explode()>, and understanding JSON processing, you can make your PHP applications more dynamic and efficient. As you proceed in your journey to become a web developer, remember that experimenting with code and exploring PHP’s built-in functions are the best ways to deepen your understanding and proficiency. Happy coding!