Control Structures and Loops in PHP: Writing Conditional and Repetitive Code
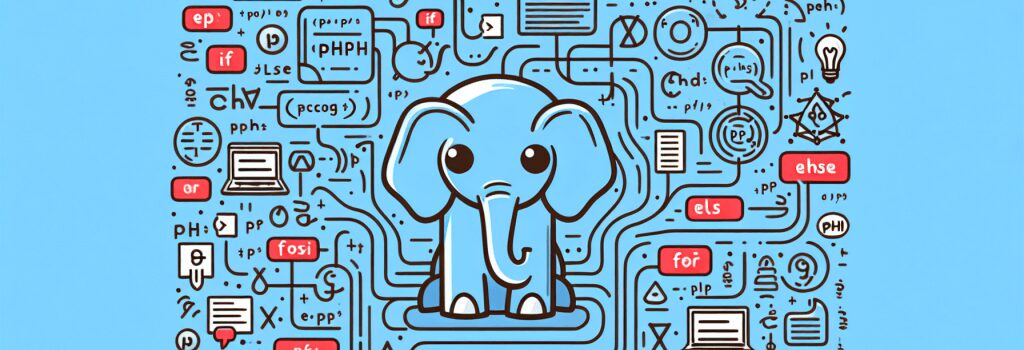
Understanding Control Structures and Loops in PHP
Programming with Conditionals: If-Else Constructs
In PHP, control structures are the cornerstone of dynamic applications. They allow your programs to make decisions and execute code blocks conditionally. The ;if> statement is among the most used control structures, enabling your code to branch based on conditions.
This simple example demonstrates how PHP decides what code to execute based on the condition provided. Enhancements can be made using ;elseif> to handle multiple conditions effectively.
Switch Statements for Multiple Conditions
For scenarios where multiple conditions lead to different outcomes, the ;switch> statement provides a cleaner syntax compared to multiple ;if-elseif> statements.
Looping Through Code with For, While, and Foreach
Repetitive tasks are common in programming. PHP offers several looping structures to handle these situations efficiently.
The For Loop
The ;for> loop is ideal for iterating a set number of times. It consists of an initializer, a condition, and an incrementor.
The While Loop
The ;while> loop keeps running as long as its condition is true. It’s suitable for situations where the number of iterations isn’t predetermined.
The Foreach Loop
The ;foreach> loop is tailor-made for arrays, providing an easy way to iterate over each element.
Combining Loops and Conditional Statements
Real-world problems often require the combination of loops and conditional statements. This approach allows for more complex and dynamic applications.
Conclusion
Control structures and loops are fundamental in PHP, enabling developers to write dynamic, efficient, and readable code. By mastering these constructs, you’ll be well on your way to developing complex web applications. Remember, practice is key, so experiment with different control structures and loops to deepen your understanding.