Connecting PHP to MySQL: A Beginner’s Tutorial
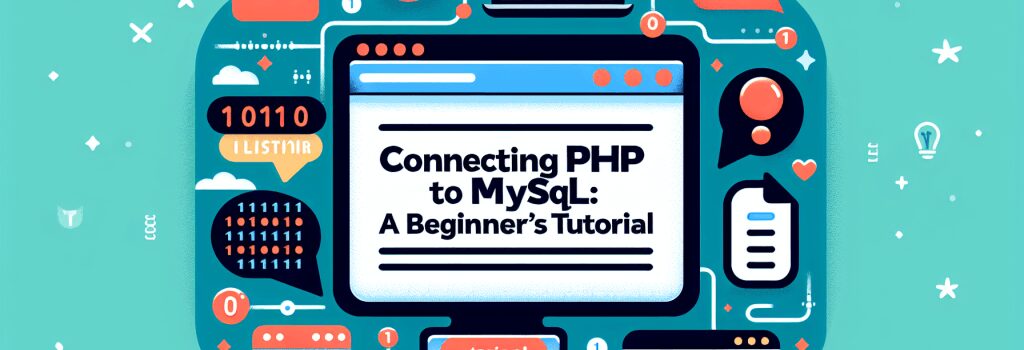
—As the world of web development continues to evolve, the need for dynamic, data-driven websites has become ever more prevalent. For those just starting out in web development, mastering the interaction between PHP (Hypertext Preprocessor) and MySQL databases can be a pivotal skill. This beginner’s guide will walk you through the essential steps of connecting PHP to a MySQL database, enabling you to start building dynamic web applications.
Understanding PHP and MySQL
Before diving into code, it’s important to understand the roles PHP and MySQL play in web development. PHP is a server-side scripting language designed specifically for web development. It allows developers to create dynamic content that interacts with databases. MySQL, on the other hand, is a database management system that uses SQL (Structured Query Language) to manage and manipulate databases.
Setting Up Your Environment
To start working with PHP and MySQL, you must have a server environment set up. You can do this by installing software packages like XAMPP, WAMP, or MAMP on your computer. These packages include Apache server, MySQL, and PHP, providing you with a local development environment.
Installing a Local Server Environment
1. Download and Install XAMPP/WAMP/MAMP from their respective websites.
2. Follow the installation instructions.
3. Start the modules Apache and MySQL.
Now, you have a functioning local server environment ready for PHP and MySQL development.
Connecting PHP to MySQL
With your local server environment set up, you’re now ready to connect PHP to MySQL. The connection process involves using PHP functions to communicate with the MySQL database.
PHP Code to Connect to MySQL
Here is a simple script to connect PHP to a MySQL database:
Explanation of the Code
– ;$servername> is normally set to "localhost" when you’re working on a local server.
– ;$username>, ;$password>, and ;$dbname> should be replaced with your database’s username, password, and name, respectively.
– The ;mysqli> object is created and used to establish the connection.
– The ;connect_error> function checks if the connection was successful and prints an error message if it wasn’t.
Creating a Database and Table
To store and manage your data, you need to create a database and at least one table.
SQL Query to Create a Database
SQL Query to Create a Table
Inserting Data into the Table
Once you have a table, you can start inserting data into it using PHP.
Retrieving Data from the Table
To display data from your database on a webpage, you can use a SELECT query.
Conclusion
Connecting PHP to a MySQL database is a fundamental skill for any aspiring web developer. Through this tutorial, you’ve seen how to set up a local development environment, establish a connection between PHP and MySQL, and perform basic database operations such as creating a database, inserting, and retrieving data. With these basics under your belt, you’re well on your way to developing dynamic web applications.
Remember, practice is key. Don’t hesitate to experiment with different queries and PHP functions to broaden your understanding and skills.
—This guide is meant to serve as a starting point. As you grow more comfortable with these concepts, you’ll find there’s much more to learn and explore in the realm of PHP and MySQL web development. Happy coding!