Advanced Object-Oriented Programming Concepts in PHP
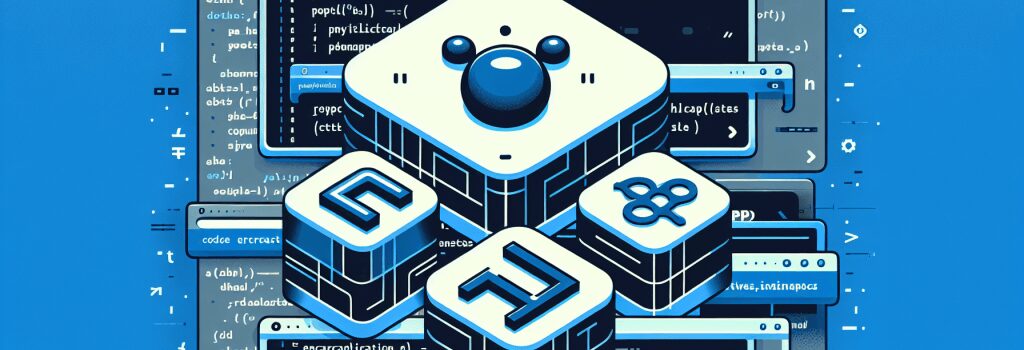
Harnessing the Power of Advanced Object-Oriented Programming in PHP
Object-Oriented Programming (OOP) stands at the core of modern PHP development, allowing developers to create more flexible, efficient, and manageable code. By leveraging advanced OOP concepts, you can elevate your PHP applications to new heights, ensuring scalability and robust architecture. This comprehensive guide dives deep into the complexities of object-oriented programming in PHP, providing you with the tools and knowledge to master advanced techniques.
Understanding Object Classes and Inheritance
At its heart, OOP in PHP revolves around the creation and manipulation of objects—a self-contained bundle of variables and functions, known as properties and methods, respectively.
Encapsulation: The Building Block of OOP
Encapsulation is a fundamental principle of OOP that restricts direct access to an object’s components. In PHP, visibility keywords like ;public>, ;protected>, and ;private> control access levels, ensuring that an object’s internal state cannot be altered unexpectedly.
Leveraging Inheritance
Inheritance allows a class to use properties and methods of another class. In PHP, this is accomplished with the ;extends> keyword, creating a parent-child relationship where child classes inherit from their parents, promoting code reuse and efficiency.
Polymorphism: Beyond Basic Inheritance
Polymorphism, or the ability of different classes to respond to the same message in unique ways, is another cornerstone of OOP. It enriches PHP applications by providing flexibility in how objects interact.
Interfaces and Abstract Classes
To implement polymorphism in PHP, interfaces and abstract classes are pivotal. Interfaces declare methods that all implementing classes must adhere to, while abstract classes allow you to define both concrete and abstract methods.
Advanced OOP Features in PHP
PHP’s OOP model includes several advanced features that enhance code modularity and reusability.
Traits
Traits are a mechanism for code reuse in single inheritance languages like PHP. They allow developers to create groups of methods that can be inserted into classes, reducing code duplication.
Namespaces
As PHP applications grow, managing classes and preventing name conflicts become crucial. Namespaces provide a way to encapsulate related classes, interfaces, and functions, making large applications more manageable.
Late Static Bindings
Late static bindings enable a class to reference itself in a context-appropriate way. It is particularly useful in inheritance hierarchies, where ;static> methods need to refer to the called class rather than where the method is defined.
Conclusion
Mastering advanced OOP concepts in PHP is essential for developing high-quality, maintainable web applications. By understanding and implementing principles like encapsulation, inheritance, and polymorphism, utilizing abstract classes, interfaces, traits, namespaces, and late static bindings, you can harness the full power of PHP to create versatile and efficient applications. As you continue to explore these advanced concepts, remember that the essence of OOP lies in creating code that is not just functional but also elegant and easy to manage.