Working with JSON in PHP: Arrays and Strings
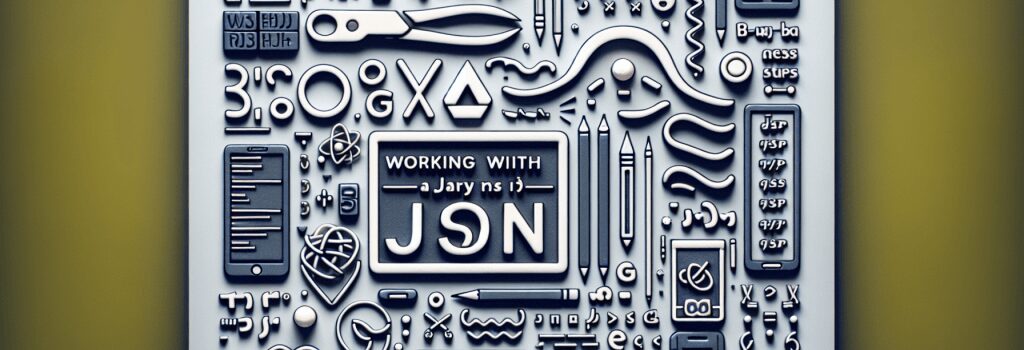
Understanding JSON in PHP: Handling Arrays and Strings
When delving into the world of Backend Development with PHP, one crucial skill set you need to master is working with JSON (JavaScript Object Notation). JSON is a lightweight data-interchange format that is easy for humans to read and write and for machines to parse and generate. It is based on a subset of JavaScript Programming Language, making it highly relevant for web developers. This guide will take you through how to effectively work with JSON in PHP, focusing on arrays and strings, helping you become proficient in handling web data.
JSON and PHP: The Basics
Before diving into the specifics, it’s essential to understand what JSON is. JSON is a text format that facilitates data exchange between a server and a client or between server-side scripts. In the context of PHP, JSON plays a pivotal role in web applications, enabling the seamless transfer of data from the server to the client side, and vice versa.
Encoding Arrays and Strings in JSON
Encoding Arrays
PHP provides a straightforward function, ;json_encode()>, for converting arrays and strings into JSON format. To encode an associative array into JSON, follow these steps:
php
$myArray = array("name" => "John", "age" => 30, "city" => "New York");
$jsonEncodedData = json_encode($myArray);
This code snippet converts the PHP associative array into a JSON string. The ;json_encode()> function processes the array and generates a string that represents the equivalent JSON object.
Encoding Strings
Similarly, encoding a simple string into JSON format involves using the same ;json_encode()> function. For instance:
php
$myString = "Hello, World!";
$jsonEncodedString = json_encode($myString);
In this example, the PHP string is converted into a JSON-formatted string. It’s worth noting that while encoding simple strings may not seem beneficial at first, it becomes crucial when dealing with complex data structures or passing data in web applications.
Decoding JSON into PHP Arrays and Strings
Decoding JSON data into PHP is as straightforward as encoding it. The ;json_decode()> function is used for this purpose. It converts a JSON-formatted string into a PHP variable (arrays or objects).
Decoding into Arrays
To convert a JSON string back into a PHP array, use ;json_decode()> with its second parameter set to ;true>:
php
$jsonString = '{"name": "John", "age": 30, "city": "New York"}';
$phpArray = json_decode($jsonString, true);
This operation transforms the JSON string into an associative array, allowing you to access its elements like any other PHP array.
Decoding into Objects
If you prefer working with objects, omit the second parameter when using ;json_decode()>:
php
$jsonString = '{"name": "John", "age": 30, "city": "New York"}';
$phpObject = json_decode($jsonString);
This code snippet converts the JSON string into a PHP object, which you can access using the ;->> syntax.
Best Practices for Working with JSON in PHP
1. Validate JSON Strings: Always validate JSON strings using ;json_last_error()> to ensure you’re working with well-formed data.
2. Avoid Deep Nesting: Deeply nested JSON structures can be complex to navigate and manage. Keep your data structure simple for easier manipulation.
3. Use JSON for APIs: JSON is the de facto standard for most web APIs. Familiarize yourself with both generating and consuming JSON data in your PHP applications.
Conclusion
Mastering JSON in PHP is a fundamental skill for any web developer, especially when working with web applications and APIs. By understanding how to encode and decode arrays and strings between PHP and JSON, developers can efficiently manage data interchange and application state, paving the way for more dynamic and responsive web experiences. Keep practicing these techniques, and you’ll find yourself adept at handling JSON data in your PHP projects.