Implementing Recursion in PHP for Advanced Problem Solving
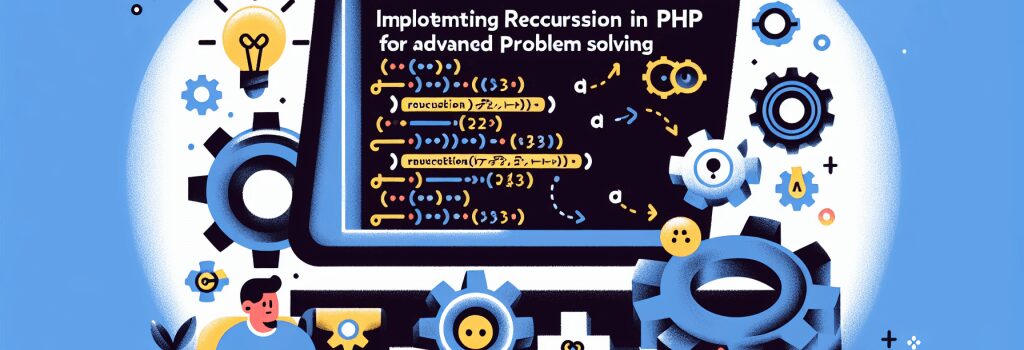
In the realm of Backend Development with PHP, mastering control structures and functions is paramount. One powerful concept that can significantly elevate your problem-solving skills is recursion. Implementing recursion in PHP can not only streamline your code but also tackle complex problems with elegance and efficiency. This article delves into the fundamentals of recursion in PHP, providing practical examples and tips to leverage this advanced programming technique effectively.
Understanding Recursion in PHP
Recursion occurs when a function calls itself directly or indirectly, allowing the execution of repetitive tasks without the clutter of loop constructs such as ;for> or ;while>. The key to avoiding infinite loops and ensuring successful recursion is to include a base case – a condition under which the function stops calling itself and returns a result.
The Anatomy of a Recursive Function
A well-structured recursive function in PHP typically involves two main components:
– Base Case: The condition under which the recursion ends.
– Recursive Case: The part of the function where the recursion occurs.
php
function recursiveFunction($param) {
if ($param == baseCaseCondition) {
return $baseCaseResult;
} else {
return recursiveFunction($modifiedParam);
}
}
Practical Example: Calculating Factorials
To fully grasp recursion’s power, let’s implement a classic example – calculating the factorial of a number. The factorial of a number ;n> (denoted as ;n!>) is the product of all positive integers less than or equal to ;n>.
php
function factorial($n) {
if ($n <= 1) {
return 1; // Base case: factorial of 0 or 1 is 1.
} else {
return $n * factorial($n - 1); // Recursive case
}
}
echo factorial(5); // Outputs: 120
Advantages of Using Recursion
Simplifies Complex Problems
Recursion breaks down complex problems into simpler or smaller instances of the same problem, thereby simplifying the code logic and making it easier to understand and debug.
Cleaner Code
By using recursion, you can write cleaner and more concise code, eliminating the need for cumbersome loop constructs and multiple temporary variables.
Tips for Implementing Recursion
– Identify the Base Case: Before diving into writing a recursive function, clearly define the condition(s) under which the recursion should terminate.
– Ensure Progress Towards the Base Case: Each recursive call should bring the execution closer to the base case to prevent infinite loops.
– Test with Simple Cases: Start testing your recursive functions with simple input values to ensure they handle base cases correctly before scaling to more complex scenarios.
Conclusion
Implementing recursion in PHP is a testament to the language’s flexibility and power in solving complex problems with succinct and elegant code. By understanding and applying recursion, you can enhance your problem-solving skills and tackle challenging tasks with greater ease. Remember, mastering recursion takes practice and patience, so take your time to experiment with different problems and recursive approaches.