Advanced Form Handling and Validation with PHP
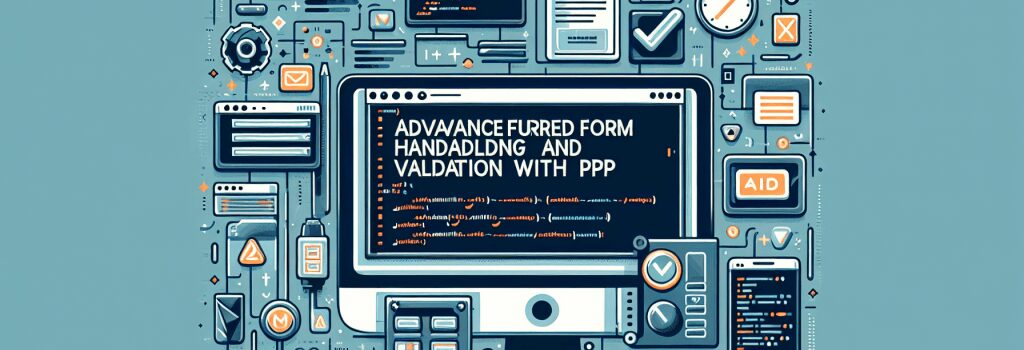
Creating dynamic web applications often involves interacting with user input, which is predominantly handled through forms. Proper handling and validation of form data in PHP not only improves the usability of your application but also secures it against malicious attacks. This guide delves deep into advanced form handling and validation techniques with PHP, ensuring your web applications are robust and user-friendly.
Understanding PHP Form Handling
Before diving into advanced concepts, it’s essential to have a strong foundation in how PHP processes form data. The superglobals ;$_GET> and ;$_POST> are utilized to collect data submitted via forms, depending on the form’s method attribute.
Processing User Input
When a user submits a form, PHP allows you to access this data using the aforementioned superglobals. For a form submitted via the GET method, use ;$_GET> to access the data; for POST, use ;$_POST>. It’s crucial to sanitize and validate this data before using it in your application to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks.
Advanced Form Validation Techniques
Form validation is a critical aspect of web development. It ensures that the data received from the user meets the application’s requirements before being processed or stored in a database.
Using filter_var() for Validation
PHP’s ;filter_var()> function is a versatile tool for validating and sanitizing data. It can validate email addresses, URLs, IP addresses, and much more. For instance, to validate an email address, you can use:
php
$email = filter_var($email, FILTER_VALIDATE_EMAIL);
if (!$email) {
echo "The email address is not valid!";
}
Regular Expressions for Custom Validation
Sometimes, pre-defined validation filters are not enough. In such cases, regular expressions (regex) come in handy. PHP’s ;preg_match()> function allows you to define complex patterns that the user input must match. This is particularly useful for phone numbers, passwords, and other inputs requiring specific formats.
Implementing CSRF Protection
Cross-Site Request Forgery (CSRF) is a security threat where unauthorized commands are transmitted from a user that the web application trusts. To counteract this, a common strategy involves adding a CSRF token in your forms. The token is a random string, stored in the user session and included as a hidden field in the form. Upon form submission, the submitted token is compared with the session token to ensure authenticity.
php
session_start();
if (empty($_SESSION['csrf_token'])) {
$_SESSION['csrf_token'] = bin2hex(random_bytes(32));
}
In the form:
html
<form method="post" action="process.php">
<input type="hidden" name="csrf_token" value="<?php echo $_SESSION['csrf_token']; ?>">
<!-- Form fields -->
</form>
Advanced Form Handling in PHP
Beyond validation, form handling in PHP can be extended to create more dynamic and interactive web applications.
AJAX and PHP
AJAX (Asynchronous JavaScript and XML) allows web pages to be updated asynchronously by exchanging data with a server in the background. This means you can validate form inputs or load new form elements without reloading the page, enhancing the user experience. Integrating AJAX with PHP backend logic requires a solid understanding of both JavaScript (or frameworks/libraries like jQuery) and PHP.
File Uploads
Handling file uploads is another critical aspect of form handling. PHP’s ;$_FILES> array provides an easy way to access uploaded files. Validating file size, type, and ensuring secure storage are all important considerations when allowing file uploads.
Conclusion
Advanced form handling and validation are integral to developing secure and user-friendly web applications. By leveraging PHP’s built-in functions, such as ;filter_var()> and ;preg_match()>, along with implementing CSRF protection and understanding AJAX integration, developers can create robust web applications. Always remember, the key to advanced form handling is not just about collecting data but doing so securely and efficiently.