Secure User Authentication: Best Practices in PHP
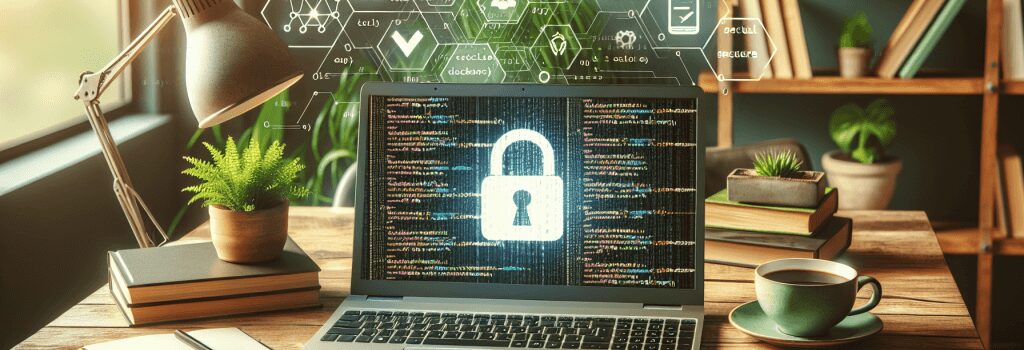
Understanding User Authentication in PHP
User authentication is a critical component in the security of web applications. It ensures that only authenticated users can access certain resources. PHP, being a widely used server-side scripting language for web development, offers various ways to implement secure user authentication practices. This article will guide you through the best practices to follow for securing user authentication in your PHP applications.
Secure Hashing Algorithms
Use Up-to-date Hashing Functions
It’s crucial to store user passwords securely. PHP provides functions like ;password_hash()> and ;password_verify()> to hash and verify passwords safely. Always use ;PASSWORD_DEFAULT> as the algorithm since PHP will automatically use the latest and most secure hashing algorithm available.
Avoid MD5 and SHA1
Older hashing algorithms like MD5 and SHA1 are no longer considered secure due to their vulnerability to collision attacks. Always opt for more secure options such as bcrypt, which is currently implemented by PHP’s ;password_hash()> function.
Implementing Two-factor Authentication (2FA)
Add an Extra Layer of Security
Two-factor Authentication (2FA) significantly enhances your application’s security by requiring another form of verification in addition to the password. This can be an SMS code, an email, or an authenticator app. Implementing 2FA protects against compromised passwords and adds a critical security layer to user authentication.
Secure Session Management
Regenerate Session IDs
Always regenerate a new session ID using ;session_regenerate_id()> whenever the privilege level changes, such as upon logging in. This prevents session fixation attacks, where an attacker could use a predefined session ID to gain unauthorized access.
Use Secure Cookies
Set cookies with the Secure and HttpOnly flags to true. The Secure flag ensures cookies are sent over HTTPS, reducing the risk of man-in-the-middle attacks. The HttpOnly flag prevents client-side scripts from accessing the data, safeguarding against cross-site scripting (XSS) attacks.
Protect Against Common Threats
Guard Against SQL Injection
Use prepared statements with bound parameters to prevent SQL injection, one of the most common security vulnerabilities in web applications. PHP’s PDO (PHP Data Objects) or MySQLi extension can be used to prepare statements that are both safe and efficient.
Prevent Cross-Site Scripting (XSS)
Always sanitize and validate user inputs to protect against XSS attacks. Use functions like ;htmlspecialchars()> to encode special characters from the input data, preventing the execution of malicious scripts.
Cross-Site Request Forgery (CSRF) Protection
A CSRF attack forces a logged-in user to perform unintended actions. Use anti-CSRF tokens in your forms and validate them upon submission to mitigate this risk.
Conclusion
Securing user authentication in PHP applications encompasses various strategies, from using robust hashing algorithms to implementing 2FA and securing sessions and cookies. By adhering to these best practices, developers can significantly reduce the risk of unauthorized access and information disclosure, building more secure web applications for their users. Always keep security at the forefront of your development process to safeguard your applications and user data against emerging threats.