Cross-Site Scripting (XSS) Prevention in JavaScript
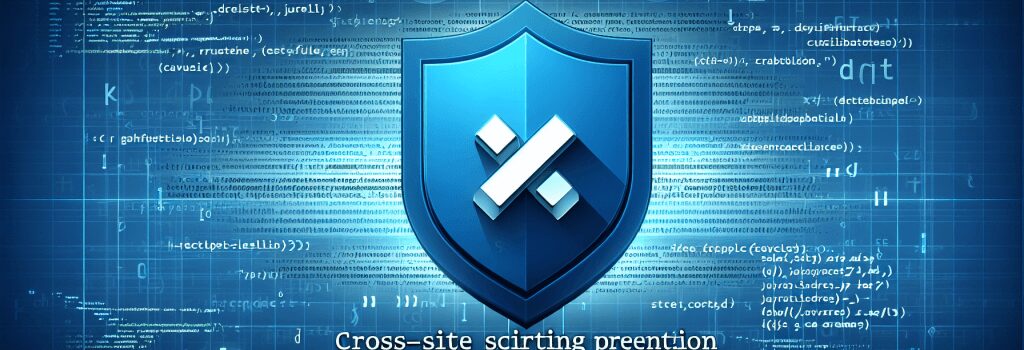
Understanding Cross-Site Scripting (XSS) and Its Importance in Web Development
Cross-Site Scripting (XSS) is a prevalent security vulnerability that affects many websites, posing a significant threat to both users and organizations. By injecting malicious scripts into web applications, attackers can gain unauthorized access to user data, compromise user sessions, and perform actions on behalf of users without their consent. For web developers, understanding XSS and employing strategies to mitigate this vulnerability is not just recommended—it’s imperative.
What is Cross-Site Scripting?
XSS attacks occur when an attacker finds a way to insert malicious scripts into web content that is viewed by other users. These scripts can then execute in the context of the user’s session, allowing attackers to steal cookies, session tokens, or even sensitive information displayed on the page.
Types of XSS Attacks
1. Stored XSS: The malicious script is permanently stored on the target server.
2. Reflected XSS: The script is reflected off the web server through an error message, search result, or any other response that includes some or all of the input sent to the server.
3. DOM-based XSS: The vulnerability exists in the client-side code rather than the server-side code.
XSS Prevention Techniques in JavaScript
Validate and Sanitize Input
Validating and sanitizing user input is the first line of defense against XSS attacks.
– Validation: Ensure that the input matches the expected format, length, and type.
– Sanitization: Remove or encode potentially dangerous characters from the input.
Use Text Content Properly
When dynamically setting the content of web elements, it’s crucial to do so safely.
– Use ;.textContent> instead of ;.innerHTML> when inserting text into the DOM. This prevents the browser from interpreting the content as HTML, thus avoiding the execution of any embedded scripts.
Leverage Content Security Policy (CSP)
Implementing a Content Security Policy (CSP) can significantly reduce the risk of XSS attacks. CSP is a browser feature that allows you to specify trusted sources of content and restricts the browser from loading any resources from untrusted sources.
– Define a CSP policy in the web server’s headers to control the sources from which scripts can be loaded.
Utilize Modern JavaScript Frameworks
Modern JavaScript frameworks like React, Angular, and Vue.js have built-in XSS protections that automatically escape HTML. By using these frameworks for web development, you inherently reduce the risk of XSS vulnerabilities.
Encode Data
When inserting untrusted data into HTML, JavaScript, CSS, or URL contexts, encode the data according to the context:
– HTML entities encoding for data inserted into HTML.
– JavaScript encoding for data inserted into JavaScript.
– URL encoding for data inserted into URLs.
Regularly Update and Audit Your Code
Maintain regular updates and security audits of your codebase. This includes keeping any libraries or frameworks you are using up-to-date, as they may include patches for known vulnerabilities.
Conclusion
Preventing XSS attacks requires vigilance and a proactive approach to web development. By understanding XSS attacks and implementing the practices outlined above, developers can significantly enhance the security of their web applications. Remember, security is not a one-time task but a continuous process of improvement and adaptation to new threats. Protect your users, protect your data, and maintain the integrity of your web applications by prioritizing XSS prevention.