Working with Attributes and Classes in JavaScript
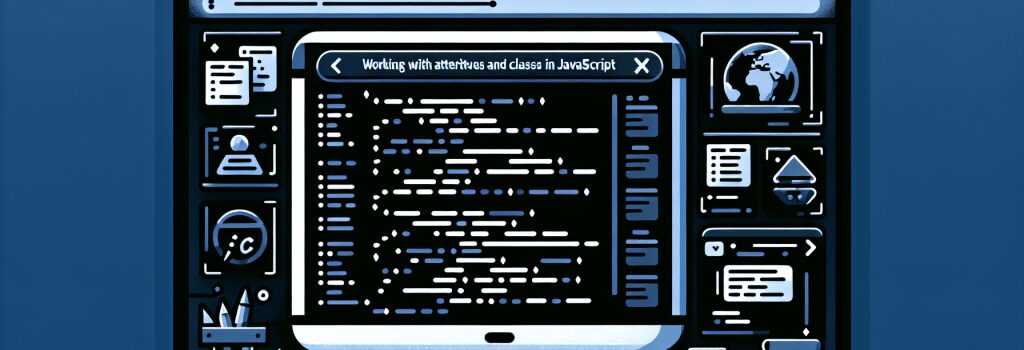
Understanding Attributes and Classes in JavaScript
JavaScript is a powerful programming language that is widely used for web development. One of the key features of JavaScript is its ability to interact with HTML elements, allowing developers to dynamically manipulate web pages. In this section, we will delve into the intricacies of working with attributes and classes in JavaScript, focusing on how to access, add, change, and remove them.
The Importance of Attributes and Classes
Attributes and classes are fundamental to HTML, providing additional information about elements. Attributes define characteristics like IDs, classes, source URLs for images, href values for links, and much more. Classes, on the other hand, are a special attribute used to categorize elements, enabling developers to style multiple elements simultaneously and manipulate them with JavaScript.
Accessing Element Attributes with JavaScript
To work with attributes in JavaScript, you first need to access the elements. This can be accomplished using methods like ;getElementById()>, ;getElementsByClassName()>, ;getElementsByTagName()>, or the more versatile ;querySelector()> and ;querySelectorAll()>.
Once you have accessed the element, you can use the ;getAttribute()> method to fetch the value of a specific attribute. For example:
This code snippet retrieves the ;href> attribute value from an element with the ID ;myLink>.
Modifying Attributes
To change the value of an attribute, use the ;setAttribute()> method. It requires two parameters: the name of the attribute and the new value. Here is an example:
This code changes the ;href> attribute of the element with ID ;myLink> to a new URL.
Working with Classes in JavaScript
Classes can be manipulated using the ;classList> property, providing a more intuitive interface to work with classes on elements.
Adding and Removing Classes
The ;classList> property has methods like ;add()> and ;remove()> for adding and removing classes. Here’s how you use them:
This code snippet adds the class ;new-class> and removes the class ;old-class> from the element with the ID ;myElement>.
Toggling Classes
The ;classList> property also offers a ;toggle()> method, which adds a class if it does not exist or removes it if it does. It’s particularly useful for implementing interactive elements like dropdowns:
Conclusion
Manipulating attributes and classes with JavaScript is a fundamental skill for web developers. It allows for dynamic changes to elements, enhancing interactivity and user experience. By understanding and utilizing methods like ;getAttribute()>, ;setAttribute()>, and the ;classList> property, developers can efficiently modify HTML elements to meet the needs of modern web applications. Practice working with these features to build more dynamic and responsive websites.
By mastering these techniques, you pave your way to becoming a proficient web developer, capable of creating compelling and interactive web experiences.