Variables in JavaScript: Definition and Usage
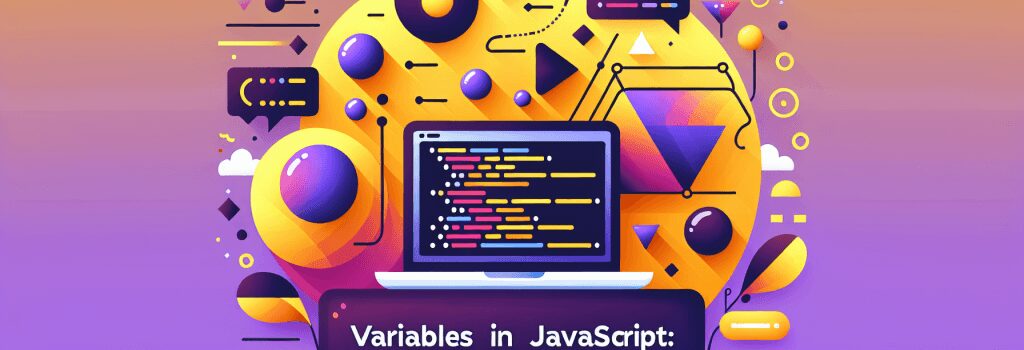
Understanding the concept of variables is fundamental in any programming language, and JavaScript is no exception. Variables are essentially containers for storing data values. In JavaScript, a variable can be declared using ;var>, ;let>, or ;const> keywords. This article delves into what variables are, their types, how to declare them, and best practices for their use in JavaScript programming.
What are Variables in JavaScript?
Variables can be thought of as named containers that you can use to store data. This data can be numbers, text (string values), or even complex objects. The importance of variables becomes evident when there is a need to reuse data throughout your JavaScript code. Instead of repeating the same value, you can store it in a variable and reference the variable instead.
Declaring Variables in JavaScript
JavaScript offers three distinct ways to declare a variable: ;var>, ;let>, and ;const>.
– Using ;var>: This is the oldest way to declare variables in JavaScript. Variables declared with ;var> are scoped to the function in which they are defined, or if declared outside any function, they become globally scoped.
– Using ;let>: Introduced in ES6 (ECMAScript 2015), ;let> allows you to declare block-scoped variables. Unlike ;var>, variables declared with ;let> are limited in scope to the block, statement, or expression in which they are used.
– Using ;const>: Also introduced in ES6, ;const> is used to declare variables whose values are meant to remain constant throughout the script. A variable declared with ;const> cannot be reassigned.
Types of Variables
In JavaScript, variables can hold various types of data, such as:
– Numbers: Both integer and floating-point numbers.
– Strings: A sequence of characters used to represent text.
– Booleans: A truthy (;true>) or falsy (;false>) value.
– Objects: Complex data structures like arrays, functions, and objects themselves.
Best Practices for Using Variables
1. Choose meaningful names: Variable names should be descriptive and hint at the type of data they hold.
2. Use ;let> and ;const> instead of ;var>: This is to avoid issues related to scope and reassignments.
3. Declare variables at the top: This makes your code cleaner and easier to understand.
4. Use camelCase for variable names: This is a widely adopted naming convention in JavaScript.
By adhering to these guidelines, developing and maintaining JavaScript code becomes much more manageable.
Conclusion
Variables in JavaScript play a crucial role in the development of dynamic and interactive web applications. Understanding the differences between ;var>, ;let>, and ;const>, as well as following best practices for variable usage, is essential for any aspiring web developer. Remember, the efficiently you use variables, the cleaner and more optimized your JavaScript code will be. Embrace the power of variables, and let them lead the way to more efficient and effective JavaScript programming.