Understanding the Basics of DOM in JavaScript
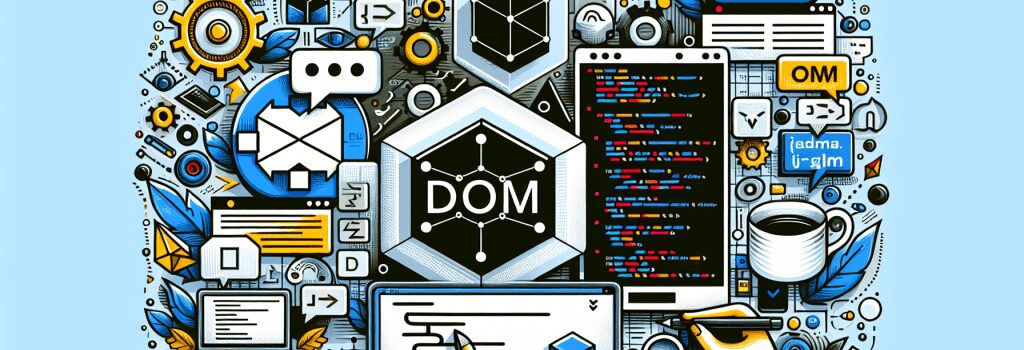
Introduction to DOM in JavaScript
The Document Object Model, or DOM, is a crucial concept for any aspiring web developer to grasp. It’s the bridge that allows JavaScript to interact with HTML and CSS, creating dynamic and interactive web experiences. In this article, we’ll dive into the basics of the DOM in JavaScript, helping you understand how it works and how you can manipulate it to bring your web pages to life.
What is the DOM?
The DOM is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as a hierarchy of nodes, where each node represents a part of the document. This could be a whole section, a paragraph, a link, or even a simple text node.
Understanding DOM Nodes
Every element in an HTML document can be accessed, changed, or removed using the DOM. The elements are organized as a tree structure, known as the “DOM Tree,” with the ;document> object at its root. From there, every document element is a node of this tree, and they are defined by their relationships with one another. For instance:
– Parent nodes: These are nodes that have other nodes (children) under them.
– Child nodes: These are contained inside another node.
– Sibling nodes: These are nodes that are at the same level or share the same parent.
How JavaScript Uses the DOM
JavaScript uses the DOM to dynamically change document content, structure, and styling. By manipulating the DOM, JavaScript can add, remove, or modify elements within the page. It can also change element styles or respond to user events, making the web page interactive.
Basic DOM Manipulation in JavaScript
To manipulate the DOM with JavaScript, you first need to access the elements you want to work with. You can do this using methods like ;getElementById()>, ;getElementsByClassName()>, ;getElementsByTagName()>, or more modern methods like ;querySelector()> and ;querySelectorAll()>.
Once you have access to an element, you can change its properties, attributes, style, or even its entire content. For example:
– Changing text content: Use the ;textContent> or ;innerText> property.
– Changing HTML content: Use the ;innerHTML> property.
– Changing CSS styles: Access the ;style> property of an element to change its CSS styling.
– Adding or removing elements: Use methods like ;appendChild()>, ;removeChild()>, or ;replaceChild()> to modify the document structure.
Event Handling in the DOM
An essential part of DOM manipulation is handling user events, like clicks, keyboard input, or mouse movements. JavaScript allows you to listen for these events on elements and execute code in response, making your page interactive.
You can attach event listeners to elements using the ;addEventListener()> method. It takes two arguments: the event you’re listening for (like ;”click”> or ;”keypress”>) and a callback function that runs when the event occurs.
Conclusion
Understanding the DOM is foundational for any web developer. By mastering how to manipulate the DOM with JavaScript, you unlock the potential to create dynamic, engaging, and interactive web experiences for your users. Remember, the key to becoming proficient in DOM manipulation is practice, so take what you’ve learned here and start applying it to your projects.
In this journey toward becoming a skilled web developer, mastering the DOM will undoubtedly be a significant milestone, empowering you to build more sophisticated and user-friendly web applications.