Understanding Control Flow in JavaScript: An Introduction
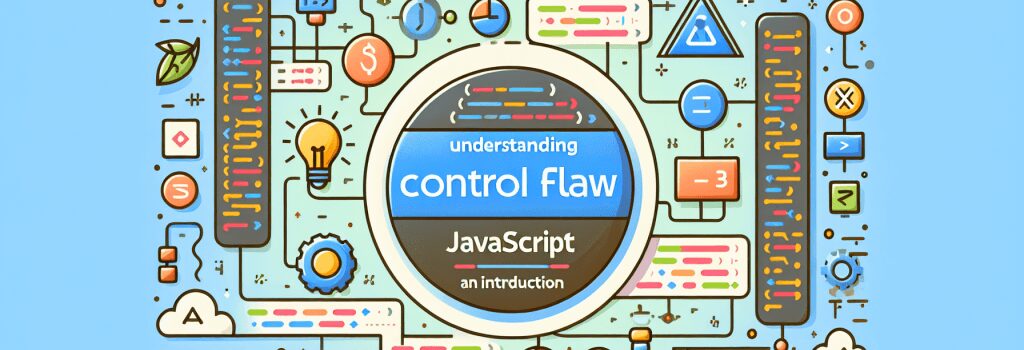
Understanding how control flow works in JavaScript is crucial for every web developer, from the beginners who are just starting out to the seasoned professionals looking to brush up on their foundational knowledge. This topic lays the groundwork for creating more dynamic and interactive web pages, empowering developers to write code that can make decisions and execute different branches based on certain conditions. In this guide, we’ll delve into the basics of control flow in JavaScript, helping you to grasp the concepts of conditional statements and how they can be wielded to bring your web applications to life.
What is Control Flow?
At its core, control flow refers to the order in which individual statements or instructions are executed or evaluated in a script. In JavaScript, as in many programming languages, code runs from top to bottom. However, this linear execution can be altered using conditional statements and loops, allowing developers to create more complex and responsive applications.
Understanding Conditional Statements
Conditional statements are the backbone of control flow in JavaScript. They allow the code to decide to execute or skip certain sections based on specific conditions. These conditions are evaluated to either ;true> or ;false>, leading to different execution paths.
The ;if> Statement
The ;if> statement is the simplest form of control flow. It executes a block of code if, and only if, a specified condition evaluates to ;true>.
The ;else> Clause
To add another layer, the ;else> clause can be used alongside the ;if> statement. This allows for an alternative block of code to be executed if the initial condition is ;false>.
The ;else if> Statement
For more complex logic involving multiple conditions, the ;else if> statement can be introduced. It adds another condition to check before resorting to the final ;else>.
The ;switch> Statement
Another way to handle multiple possible conditions is the ;switch> statement. It is often used as an alternative to multiple ;if> statements, making the code more organized and readable.
Best Practices
When working with control flow in JavaScript, keeping your code clean and readable is important. Here are a few tips to follow:
– Use clear and descriptive names for variables.
– Keep your conditions simple and avoid overly complex expressions.
– Make use of comments to explain the purpose of certain blocks of code or conditions.
– Always test different cases to ensure your code behaves as expected under various conditions.
Mastering control flow is a fundamental step towards becoming a proficient JavaScript developer. By understanding the basics of conditional statements, you can begin to craft more dynamic, efficient, and interactive web applications. With practice, you’ll become more comfortable with these concepts, allowing you to explore more advanced JavaScript features and frameworks. Control flow is your stepping stone into the vast world of web development, where your code decisions shape the user experience.