Mastering JavaScript Functions: Parameters, Arguments, and Return Values
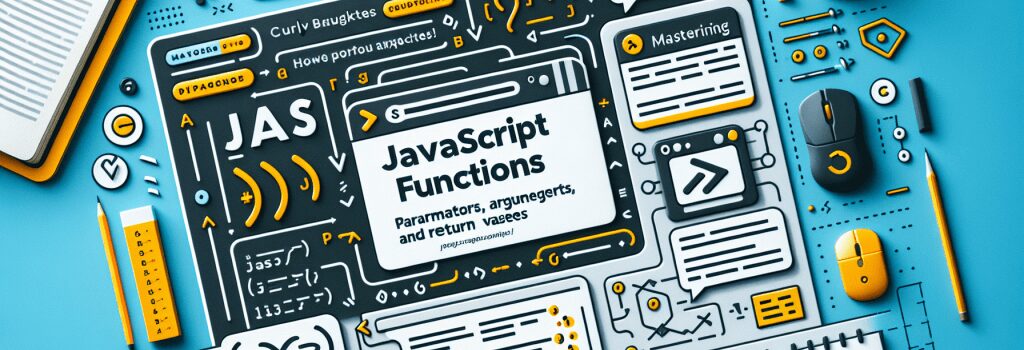
Mastering JavaScript Functions: Parameters, Arguments, and Return Values
Understanding JavaScript Functions
JavaScript functions are a cornerstone of web development, enabling programmers to write reusable code blocks that perform specific tasks. Whether you’re new to web development or looking to sharpen your JavaScript skills, a comprehensive understanding of functions, including their parameters, arguments, and return values, is essential.
What are Functions in JavaScript?
A function in JavaScript is essentially a set of instructions designed to perform a particular task. Functions are defined once and can be called multiple times, potentially with different inputs, to produce varied outcomes. This modularity and reusability make functions a powerful tool in JavaScript.
Function Parameters and Arguments
When defining or declaring a JavaScript function, you can specify parameters. Parameters are essentially placeholders for the values that a function will operate on. When a function is called, the actual values passed to it are known as arguments. Understanding the relationship between parameters and arguments is crucial for effective function design and implementation.
Parameters
Parameters are variables listed as part of the function’s definition. They represent the data that the function expects to receive when it’s called. Parameters allow functions to accept input and operate on different data without being hard-coded for specific values.
Example:
In this example, ;name> is a parameter of the function ;greet>.
Arguments
Arguments, on the other hand, are the actual values that are passed to the function when it is invoked. The arguments are what fill the placeholders provided by the parameters.
Example:
Here, ;"Alice"> is the argument passed to the ;greet> function.
Return Values in JavaScript Functions
Functions in JavaScript can also return values. A return value is the data that a function outputs after it completes its set of tasks. The ;return> statement is used to specify the value that a function should return.
The Return Statement
The return statement ends function execution and specifies a value to be returned to the function caller.
Example:
This function, ;sum>, takes two parameters, ;a> and ;b>, and returns their sum.
Functions Without Return Statements
If a function doesn’t have a return statement, it doesn’t output any value. Instead, it performs its tasks (if any) and, upon completion, implicitly returns ;undefined>.
Example:
This function displays a message but returns ;undefined> because it lacks a return statement.
Conclusion
JavaScript functions are a vital part of web development, offering the flexibility of reusing code and the efficiency of handling data-processing tasks. By mastering parameters, arguments, and return values, you can harness the full power of JavaScript functions to create dynamic, interactive, and efficient web applications.
As you continue your journey to become a web developer, keep experimenting with functions, and remember that understanding these foundational concepts is key to unlocking the potential of JavaScript and, by extension, enhancing your web development projects.