Looping with For, While, and Do-While in JavaScript
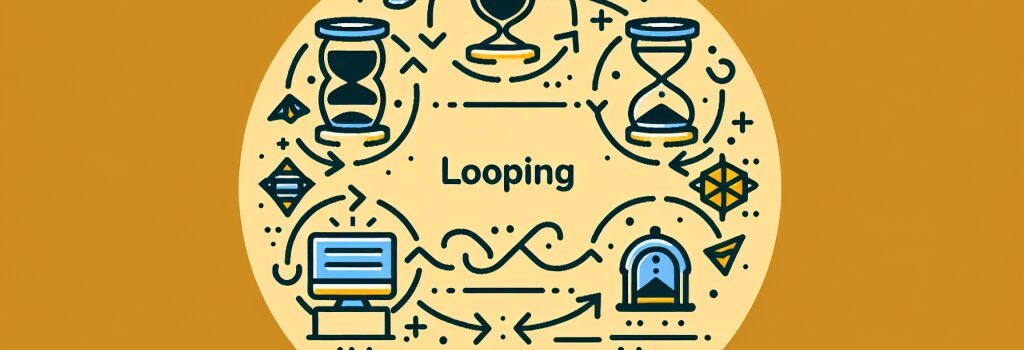
Looping structures play a crucial role in JavaScript programming, allowing developers to efficiently execute a block of code multiple times. Understanding how to use ;for>, ;while>, and ;do-while> loops is fundamental for manipulating data, handling repetitive tasks, and creating dynamic web applications. This article will explore the concepts and practical applications of these loop types to enhance your web development skills.
Understanding Looping in JavaScript
Looping in JavaScript enables developers to run the same code block repeatedly until a specified condition is met. This mechanism is not only efficient but also minimizes code redundancy, making your scripts cleaner and more readable.
For Loop
The ;for> loop is among the most commonly used looping structures in JavaScript. It is particularly useful when you know how many times you want the loop to run.
<h4>Syntax:– Initialization: Sets a variable before the loop starts (let i = 0).
– Condition: Defines the condition for the loop to run. If the condition returns true, the loop will start over again; if false, the loop will end.
– FinalExpression: Executes after each iteration of the loop, commonly used to increment the loop counter.
This loop will output numbers 0 through 4 to the console.
While Loop
The ;while> loop is suitable when you want the loop to run based on a condition, and the number of iterations is not predetermined.
<h4>Syntax:– Condition: The loop runs as long as this condition is true.
<h4>Example:This example similarly prints numbers 0 through 4, demonstrating how the ;while> loop functions.
Do-While Loop
The ;do-while> loop is a variant of the ;while> loop. The key difference is that a ;do-while> loop will always run at least once, even if the condition is false, because the condition is evaluated at the end of the loop’s block.
<h4>Syntax:This will output numbers 0 through 4, similar to the previous examples, but it’s important to note the loop’s block executes before the condition is checked.
Choosing the Right Loop
Selecting the appropriate loop type is dependent on your specific scenario:
– Use a ;for> loop when you know the number of iterations in advance.
– Opt for a ;while> loop when the iterations depend on a condition that might not be related to a counter.
– Choose a ;do-while> loop when the code block must execute at least once, but subsequent iterations depend on a condition.
Mastering loops is essential for effective JavaScript programming, particularly for tasks involving iteration over arrays, objects, or any task that requires repetitive action. By understanding and implementing ;for>, ;while>, and ;do-while> loops, you’ll be able to write more efficient, clean, and expressive code in your web development projects.