JavaScript Functions: Expressions vs Declarations
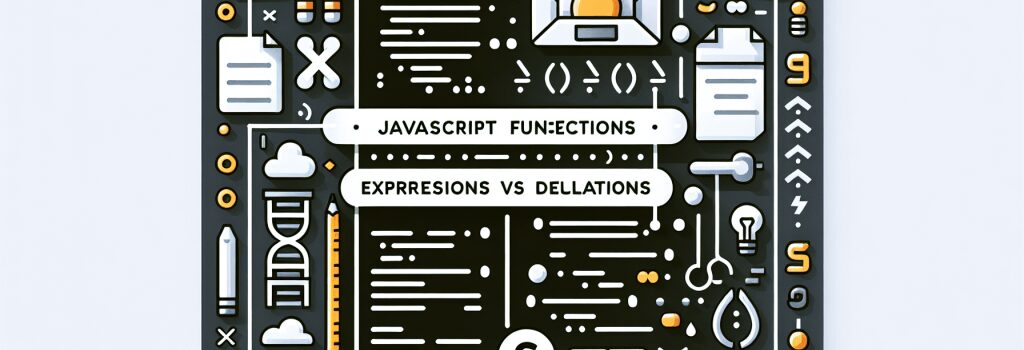
Understanding JavaScript Functions: Expressions vs Declarations
In the vast and dynamic world of web development, mastering JavaScript is crucial for anyone aiming to become an adept web developer. One fundamental concept that often puzzles beginners is the distinction between function expressions and function declarations. This article will delve into these two types of functions, elucidating their differences, usages, and how they are hoisted in JavaScript. By the end of this read, you’ll have a solid understanding of when and why to use each type.
What Are Function Declarations?
A function declaration is a traditional way of defining functions in JavaScript. It involves using the ;function> keyword followed by a unique function name, a list of parameters enclosed in parentheses, and the function body enclosed in curly braces.
Syntax
One distinguishing feature of function declarations is function hoisting, which allows you to call a function before it’s defined in the code. For example:
Despite ;sum> being called before it’s defined, JavaScript hoists the function declaration to the top of its scope, making this code work flawlessly.
What Are Function Expressions?
A function expression involves defining a function within an expression. It can be stored in a variable, and just like any other expression, it can be used as an argument to a function, as a return value, or even assigned to object properties. The function can be named, or it can be anonymous.
Syntax
Unlike function declarations, function expressions are not hoisted. This means you must define them before you use them in your code.
Function Expressions vs. Function Declarations: A Comparison
Both function expressions and declarations are pivotal in JavaScript, but their applications differ based on the need for anonymity, hoisting, and usage in code.
– Hoisting: Function declarations are hoisted, allowing them to be used before they’re defined in the execution context. Function expressions are not hoisted in this manner.
– Function Expression Anonymity: Function expressions can be anonymous, offering flexibility in scenarios like IIFEs (Immediately Invoked Function Expressions) or when assigned to variables.
– Use Cases: Function expressions are useful when passing a function as an argument to another function or when defining a function conditionally. Function declarations are straightforward and best suited for simple, top-level function definitions that need to be called from anywhere in the code.
Conclusion
Whether to use a function expression or a declaration depends on the specific requirements of your project and coding style preference. Understanding the nuances between the two, such as hoisting behavior and syntax, is critical for writing effective and error-free JavaScript. As you continue your journey to becoming a proficient web developer, mastering these concepts will undoubtedly enrich your development toolkit.
Remember, practice is key. Experiment with both types of functions in your projects to grasp their practical implications fully. Happy coding!