How to Use Fetch API for AJAX Calls in Modern JavaScript
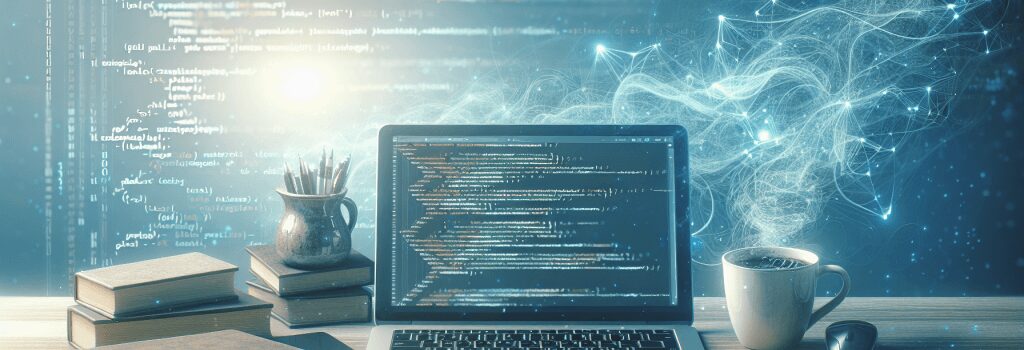
Introduction to Fetch API in Modern JavaScript
In the landscape of web development, the ability to retrieve data from a server without having to do a full page refresh has been a game-changer. This functionality, commonly referred to as AJAX (Asynchronous JavaScript and XML), has evolved significantly over the years. In modern JavaScript, the Fetch API has become the de facto standard for performing AJAX calls, offering a powerful and adaptable way to fetch resources.
Understanding the Basics of Fetch API
The Fetch API provides a global ;fetch()> method that offers an easy, logical way to fetch resources asynchronously across the network. This method returns a Promise that resolves to the Response to that request, whether it is successful or not. This marks a significant improvement over older techniques such as the XMLHttpRequest (XHR) object, as it leverages Promises for more flexible error handling and cleaner code.
Making Your First AJAX Call with Fetch
Fetching Data from a Server
Let’s kick things off with a simple example: fetching data from a server. The basic syntax for a Fetch call looks something like this:
In this snippet, we are requesting data from ;https://example.com/data>. The ;fetch()> method initiates the request and returns a promise. When the promise resolves, it returns a Response object. We then use the ;.json()> method on this response to extract the JSON body content of the response. Finally, we handle any errors that might occur during the fetch with ;.catch()>.
Handling POST Requests
The Fetch API is not just for GET requests; it can also handle POST requests. To send data to a server, you adjust the ;fetch()> call to include an options object where you specify the method, headers, and the body of your request.
Advanced Usage of Fetch API
Error Handling
A common pitfall when using Fetch is handling HTTP errors. Fetch will only reject a promise if there is a network error, but not for HTTP error statuses. You’ll need to add logic to handle such cases manually:
Working with CORS
Cross-origin resource sharing (CORS) is a mechanism that allows restricted resources on a web page to be requested from another domain outside the domain from which the first resource was served. When making cross-origin requests, it’s crucial to understand that the server needs to include specific headers to allow the request. Failure to properly handle CORS can lead to failed requests.
The Fetch API aligns with the same-origin policy, but provides an option to adjust the mode of the request to deal with CORS:
Conclusion
The Fetch API has significantly simplified the process of making AJAX calls, providing a more powerful and flexible toolset for developers. Its promise-based architecture offers a clean and readable way to handle asynchronous operations, making it a worthy addition to any web developer’s toolkit. By understanding and utilizing the Fetch API, developers can create dynamic, responsive web applications that delight users and provide seamless experiences.