Higher-Order Functions in JavaScript: Concepts and Applications
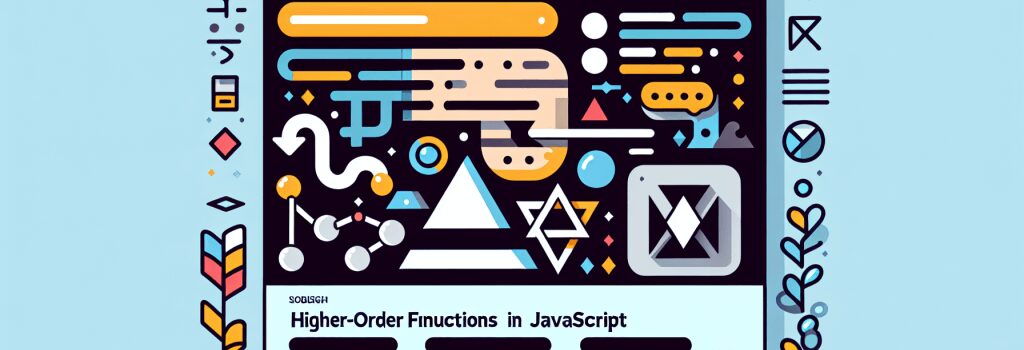
Understanding Higher-Order Functions in JavaScript
In the world of JavaScript, functions are first-class citizens. This means they can be assigned to variables, passed as arguments to other functions, and even returned from functions. A powerful concept that arises from this feature is the use of higher-order functions. These are functions that take one or more functions as arguments or return a function. By the end of this article, you will understand the concepts behind higher-order functions and learn how to leverage them in your web development projects.
What Are Higher-Order Functions?
Higher-order functions are a fundamental concept in functional programming, but they also have their place in JavaScript – a language known for its flexibility in paradigms. Essentially, any function that operates on other functions, either by taking them as arguments or by returning them, is considered a higher-order function.
Why Use Higher-Order Functions?
Higher-order functions offer several benefits:
– Abstraction and Reusability: They allow for the creation of abstractions and the encapsulation of behavior, which can lead to more reusable and maintainable code.
– Modularity: By breaking down tasks into smaller, more manageable functions, you can improve modularity in your code.
– Elegance: Higher-order functions can help in writing concise and elegant code, reducing the necessity for boilerplate.
Examples of Higher-Order Functions
<h4>Array.prototype.mapA classic example of a higher-order function is the ;map()> function, which is built into the array prototype. It takes a function as an argument and calls that function on each element of the array, returning a new array with the results.
Another example is the ;filter()> function, which returns a new array containing all elements of the original array for which the provided filtering function returns true.
Creating Your Own Higher-Order Functions
Designing your own higher-order functions can significantly increase the abstraction level of your application. Here’s a simple example of a higher-order function that takes a function as an argument and returns a new function that logs the result of the first function.
;>;javascript
function logger(fn) {
return function(…args) {
const result = fn(…args);
console.log(>Result: ${result};);
return result;
};
}
const add = (a, b) => a + b;
const loggedAdd = logger(add);
loggedAdd(2, 3);
// Output: Result: 5
>;>
Best Practices When Using Higher-Order Functions
– Readability First: Ensure that the use of higher-order functions improves, not detracts from the readability of your code.
– Avoid Overuse: While powerful, they should not be overused. Sometimes, a simple loop is more readable and efficient than using methods like ;map()> or ;filter()>.
– Performance Considerations: Keep in mind that creating many small functions can impact performance. Profile your application to identify any bottlenecks.
Conclusion
Higher-order functions are an incredibly powerful concept in JavaScript, enabling developers to write more abstract, concise, and maintainable code. By understanding and applying higher-order functions in your projects, you can exploit the full potential of JavaScript as a functional programming language. Whether you’re manipulating arrays with ;map()> and ;filter()> or creating your own higher-order functions, these tools can significantly enhance your coding toolkit.