Functions in JavaScript: Creating Reusable Code
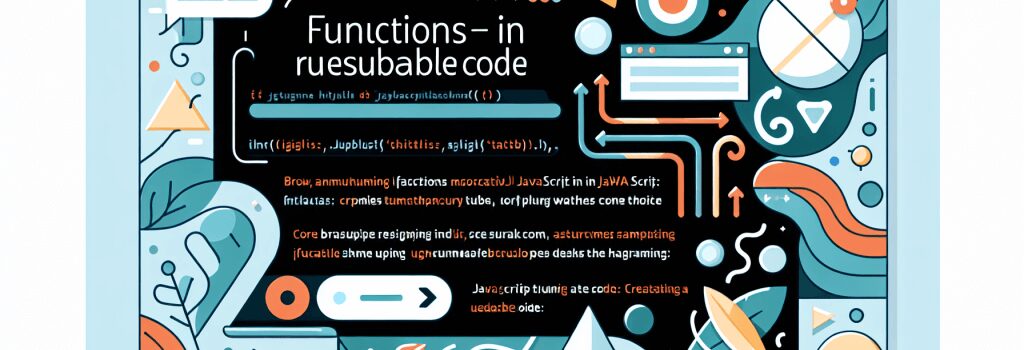
Functions in JavaScript: Embracing the Power of Reusable Code
In the realm of web development, mastering JavaScript is akin to possessing a Swiss Army knife. It’s the language that breathes life into static web pages, creating dynamic and interactive user experiences. At the heart of JavaScript’s prowess lies a powerful feature: functions. They are the building blocks that allow developers to write reusable, modular, and organized code. In this guide, we’ll explore the fundamental concepts of functions in JavaScript, guiding you through their syntax, types, and applications. So, buckle up and prepare to unlock the potential of functions, enhancing your development skills and crafting efficient code.
Introduction to Functions
Before diving into the deep end, let’s start with the basics. A function in JavaScript is essentially a set of instructions that performs a task or calculates a value. Think of it as a mini-program within your larger application. Functions are designed to be reusable: once defined, they can be invoked (called) anywhere in your program, as many times as needed.
Why Use Functions?
– Code Reusability: Functions allow you to write the code once and use it multiple times. This minimizes repetition and enhances code maintainability.
– Modularity: They enable you to divide your code into smaller, manageable units. This improves readability and makes debugging easier.
– Abstraction: Functions can hide complex operations behind simple calls, making your code cleaner and more intuitive.
Defining and Calling Functions
Basic Syntax
The simplest way to define a function in JavaScript is using the ;function> keyword, followed by the name of the function, a pair of parentheses ;()>, and a set of curly braces ;{}> where the code block is written.
To call or invoke the function, simply use its name followed by parentheses:
Parameters and Arguments
Functions become even more powerful when they can be customized each time they’re called. This is achieved through parameters and arguments.
– Parameters are variables listed as part of the function’s definition.
– Arguments are values passed to the function when it is invoked.
Types of Functions
Anonymous Functions
An anonymous function is a function without a name. These are often used as arguments of other functions or assigned to variables.
Arrow Functions
Introduced in ES6, arrow functions offer a concise syntax for writing functions. They are especially useful for short, single-action functions.
Conclusion
Functions in JavaScript are fundamental to writing clean, efficient, and maintainable code. By understanding how to define and use functions, you’re well on your way to becoming a proficient web developer. Remember, practice is key. Experiment with different types of functions and utilize them to perform various tasks in your applications.
As you continue your journey into web development, embracing the power of functions will undoubtedly open doors to creating more dynamic and interactive web experiences. Happy coding!