Exploring Object-Oriented Programming in JavaScript
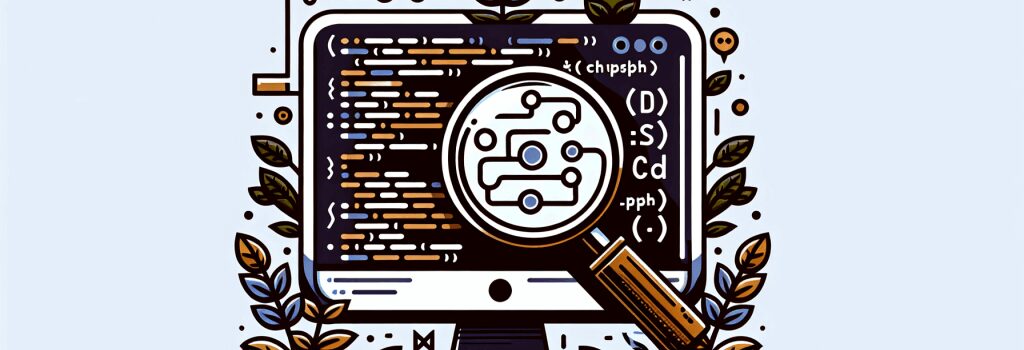
Introduction to Object-Oriented Programming in JavaScript
Object-Oriented Programming (OOP) is a programming paradigm centered around the concept of objects. These objects can contain data, in the form of fields or properties, and code, in the form of functions or methods. JavaScript, being a highly flexible programming language, supports Object-Oriented Programming, allowing developers to structure their applications in a more modular and manageable way.
Understanding Objects in JavaScript
The Basics of JavaScript Objects
In JavaScript, objects are king. If you understand objects, you understand the language. Essentially, an object is a standalone entity, with properties and type. It’s a collection of key-value pairs, where the keys are strings (or Symbols) and the values can be anything from strings to functions.
Creating an object in JavaScript is straightforward:
This simple object ;person> has two properties: ;firstName> and ;lastName>, and one method: ;greet>.
Classes in JavaScript
ECMAScript 6 introduced classes to JavaScript, offering a new syntax for creating objects and dealing with inheritance. Though it’s important to note that JavaScript classes are syntactical sugar over the existing prototype-based inheritance and do not introduce a new object-oriented inheritance model.
Defining a class in JavaScript looks like this:
Instances of classes are created in the same way as traditional functions:
Inheritance in JavaScript
One of the pillars of Object-Oriented Programming is inheritance—a mechanism that allows one class to extend another, inheriting its properties and methods.
In JavaScript, inheritance is achieved through prototypes. Every object in JavaScript has a property called ;prototype>, where you can add methods and properties to it, and every object created from this object will inherit these methods and properties.
However, with ES6 classes, inheritance is more syntactically pleasing:
;>;javascript
class Employee extends Person {
constructor(firstName, lastName, jobTitle) {
super(firstName, lastName); // Calls the parent class constructor
this.jobTitle = jobTitle;
}
describe() {
console.log(>Hello, I am ${this.firstName} ${this.lastName} and I am a ${this.jobTitle}.;);
}
}
>;>
Here, ;Employee> inherits from ;Person>, and we can add new properties or methods, or override existing ones.
Conclusion
Object-Oriented Programming in JavaScript provides a robust framework for structuring complex web applications. By understanding and utilizing objects, classes, and inheritance, developers can create more modular, reusable, and manageable code. JavaScript’s flexibility and powerful features make it an excellent choice for web development, enabling both beginners and experienced developers to master OOP concepts effectively.
Embracing the object-oriented paradigm in JavaScript will surely elevate your web development skills and open the doors to creating sophisticated web applications.