Event Handlers and Callback Functions in JavaScript
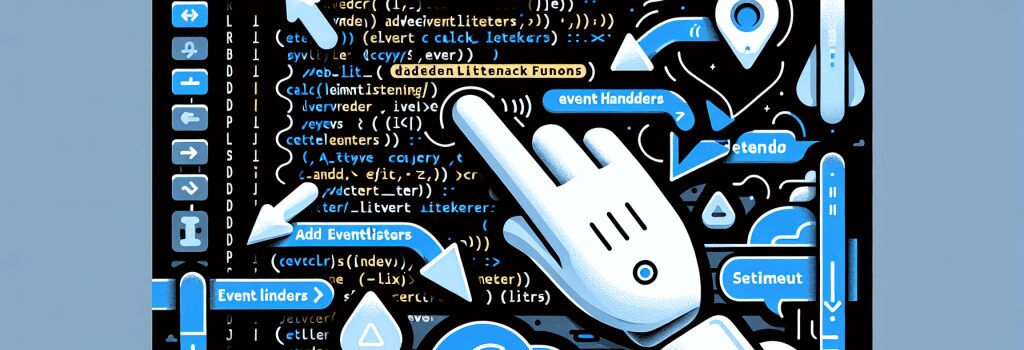
Understanding Event Handlers and Callback Functions in JavaScript
Event Handlers: The Building Blocks of Interactive Web Applications
In the realm of web development, creating interactive user experiences is paramount. JavaScript, the language of the web, provides developers with the tools needed to bring static pages to life. One of the cornerstones of this interactivity is the concept of event handlers.
Event handlers are functions in JavaScript that are executed in response to specific events occurring within the browser. These events could be anything from a user clicking a button, hovering over a link, to the loading of a web page. By leveraging event handlers, developers can craft responsive, dynamic web applications that engage and respond to user actions in real-time.
How to Work with Event Handlers
To utilize an event handler, you first need to identify the event you want to listen for. Common events include ;click>, ;mouseover>, ;mouseout>, ;keydown>, ;load>, and many more. Once the event type is determined, you bind an event handler to an element in the DOM (Document Object Model) that will listen for that event.
javascript
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
In the above example, an anonymous function is used as an event handler. This function is executed when the button with the ID ;myButton> is clicked.
Unlocking Advanced Interactivity with Callback Functions
Callback functions take the concept of event handlers a step further. A callback function is a function passed into another function as an argument, which is then invoked inside the outer function to complete some kind of routine or action.
In JavaScript, callback functions are crucial for handling asynchronous operations such as server requests, timers, and, of course, event handlers. They allow for more flexible code structures and can be used to execute a sequence of events or operations only after the previous one has completed.
How Callback Functions Enhance Event Handlers
Consider an example where you need to fetch user data from a server before displaying it on the webpage. By using a callback function with an event handler, you can ensure the data is fully loaded and processed before presenting it to the user.
javascript
document.getElementById("loadUserData").addEventListener("click", function() {
fetchUserData(function(userData) {
displayUserData(userData);
});
});
Here, ;fetchUserData> is a function that retrieves user data from a server. It takes a callback function as an argument, ;displayUserData>, which is executed only after the user data has been successfully retrieved.
Best Practices for Using Event Handlers and Callback Functions
– Keep event handlers as lean as possible. Offload heavy lifting to other functions if needed.
– Use named functions for event handlers and callbacks when possible for better readability and easier debugging.
– Be mindful of memory leaks and performance issues. Always remove event listeners when they are no longer needed.
Conclusion
Event handlers and callback functions are pivotal in creating interactive and responsive web experiences. By understanding and implementing these concepts, developers are equipped to build web applications that not only look great but also feel lively and engaging to the user. As you embark on your journey to become a skilled web developer, mastering these JavaScript foundations will undoubtedly set you on the path to success.
—This brief walkthrough of event handlers and callback functions in JavaScript aims to provide beginners with a solid starting point for deeper exploration. As you grow as a web developer, you’ll find these concepts instrumental in crafting advanced, user-centric web applications.