Error Handling in JavaScript for AJAX Requests
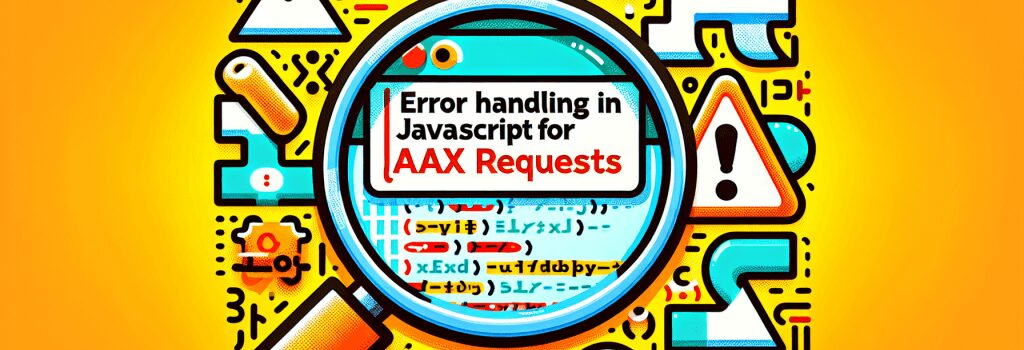
Understanding Error Handling in JavaScript for AJAX Requests
In the journey of becoming a proficient web developer, mastering JavaScript and AJAX requests is pivotal. AJAX, which stands for Asynchronous JavaScript and XML, allows for the creation of dynamic and interactive user experiences by enabling web pages to be updated asynchronously by exchanging data with a web server. However, as with any technology, things can go awry, making error handling an essential skill. This article delves into the nuances of managing errors in JavaScript when dealing with AJAX requests, ensuring that your applications are robust and user-friendly.
Why Error Handling is Vital
Error handling in JavaScript, especially for AJAX requests, is critical for several reasons. Firstly, it enhances the user experience by gracefully informing users about issues without breaking the functionality of your page. Secondly, it aids in debugging by providing clear insights into what went wrong, making it easier to identify and fix issues. Lastly, proper error handling contributes to the overall reliability and robustness of your web application, ensuring smooth operation even when faced with unexpected situations.
Types of Errors in AJAX Requests
There are primarily two types of errors you might encounter with AJAX requests:
1. Network Errors: These occur when a request fails to reach the server due to connectivity issues. It includes scenarios like server downtime or the user losing their internet connection.
2. HTTP Errors: Once the request reaches the server, it might still fail due to various reasons such as authentication failures (401), forbidden access (403), or resource not found (404). These errors are reflected in the HTTP status code of the response.
Handling Errors with XMLHttpRequest
When using the traditional ;XMLHttpRequest> object for AJAX, you can handle errors by listening to the ;error> event for network issues and checking the ;status> property of the XMLHttpRequest object for HTTP errors.
javascript
var xhr = new XMLHttpRequest();
xhr.open('GET', 'your-endpoint-url', true);
xhr.onerror = function() {
// Handle network errors
console.error("Request failed due to network error.");
};
xhr.onload = function() {
if (xhr.status >= 200 && xhr.status < 300) {
// Success logic here
} else {
// Handle HTTP errors
console.error("Server responded with HTTP error code:", xhr.status);
}
};
xhr.send();
Working with Fetch API
The ;Fetch API> offers a more modern and powerful approach to making AJAX requests. It returns promises, making it easier to work with asynchronous operations and error handling.
javascript
fetch('your-endpoint-url')
.then(response => {
if (!response.ok) {
// Handle HTTP errors
throw new Error("Server responded with HTTP error code: " + response.status);
}
return response.json();
})
.then(data => {
// Success logic here
})
.catch(error => {
// This will catch network errors and any throw statements in the .then() blocks
console.error("Failed to fetch data:", error.message);
});
Best Practices in Error Handling
– Graceful Degradation: Make sure that your application can gracefully handle errors. For instance, display user-friendly messages instead of raw error messages.
– Log Errors: Keep a log of errors. This is invaluable for debugging purposes and can help in predicting potential points of failure.
– Retry Mechanisms: For some types of errors, especially network-related, consider implementing retry mechanisms to attempt the request again.
– User Feedback: Always provide feedback to the user. If an operation fails, inform the user with a message that indicates what happened and possible next steps.
Conclusion
Mastering error handling in JavaScript for AJAX requests is a cornerstone in developing interactive and resilient web applications. By understanding the types of errors that can occur and learning how to handle them effectively, you can ensure that your application provides a seamless user experience, regardless of the circumstances. Remember, the goal is not just to fix errors, but to handle them in a way that maintains the integrity and usability of your application.