Error Handling in JavaScript: Best Practices
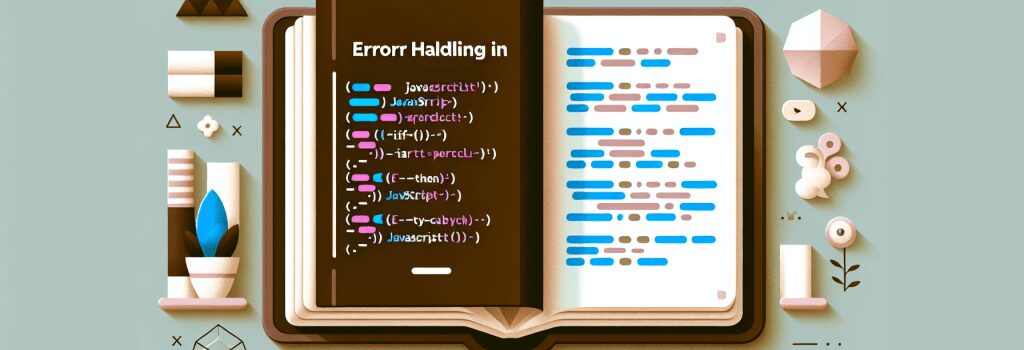
Error Handling in JavaScript: Understanding and Best Practices
As we dive deeper into the world of web development, mastering JavaScript is crucial. One key aspect that sets apart novice from experienced developers is how they approach error handling. Efficient error handling not only improves code reliability but also enhances user experience. Let’s explore the best practices for error handling in JavaScript.
Understanding Errors in JavaScript
JavaScript errors are disruptions in the execution flow of a program. They can be caused by various factors, including typos, incorrect API usage, or unforeseen user inputs. Understanding the types of errors and their causes is the first step in managing them effectively.
Syntax Errors
Syntax errors occur when the code doesn’t follow JavaScript syntax rules. These are typically caught by the JavaScript engine and reported via the console before the script is executed.
Runtime Errors
Runtime errors happen when a script is executing. They are usually caused by illegal operations, such as trying to access a property of an undefined variable.
Logical Errors
Logical errors are the hardest to detect since the code runs without crashing, but it doesn’t perform as expected. These require thorough testing and debugging to identify.
Best Practices for Handling Errors
To ensure robust error handling in JavaScript, follow these best practices:
1. Using try-catch for Error Handling
Wrap any code that might throw an error in a ;try-catch> block. This approach enables you to catch the error and decide how to handle it, possibly logging it or showing a user-friendly message.
2. Throwing Custom Errors
Sometimes, it’s beneficial to throw your errors using the ;throw> statement, especially when validating input. This allows for more granular control over error handling.
3. Leveraging finally
The ;finally> block in a ;try-catch-finally> structure executes regardless of whether an error was caught. This is ideal for cleaning up resources, such as closing files or database connections.
4. Using Promises and async-await for Asynchronous Error Handling
Modern JavaScript heavily relies on asynchronous operations, especially in web development. Promises and async/await syntax provide cleaner ways to handle errors in asynchronous code compared to traditional callback functions.
5. Logging and Monitoring Errors
While handling errors is crucial, logging them for future analysis is equally important. Keep an eye on repetitive errors or patterns that may suggest underlying issues in your application.
Conclusion
Effective error handling in JavaScript is not just about preventing the application from crashing. It’s about creating a resilient, user-friendly, and maintainable codebase. By understanding different types of errors and applying these best practices, you can significantly improve the reliability of your web applications. Remember, the goal is to anticipate potential errors, handle them gracefully, and learn from them to enhance the overall user experience.