Encapsulation and Inheritance in JavaScript: Working with Objects
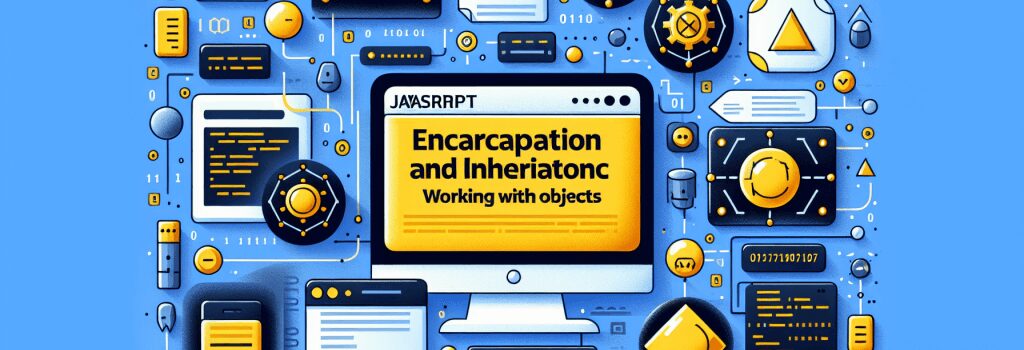
Encapsulation and inheritance are two fundamental concepts in object-oriented programming (OOP) that also play a vital role in JavaScript development. Understanding these concepts can greatly enhance your ability to write clean, efficient, and maintainable code. In this guide, we’ll delve into how encapsulation and inheritance can be utilized in JavaScript, especially when working with objects, to build more robust web applications.
Understanding Encapsulation in JavaScript
What is Encapsulation?
Encapsulation is an OOP principle that involves bundling the data (attributes) and the methods (functions) that operate on the data into a single unit or class. In JavaScript, encapsulation can be achieved using functions or the more recent class syntax introduced in ECMAScript 2015 (ES6).
Implementing Encapsulation with Functions
Before ES6, developers used functions to simulate classes and achieve encapsulation. Here’s a simple example:In this example, ;salary> is a private attribute because it’s defined within the function scope and is not accessible outside of it. The ;getSalary> method is public and is used to access the private ;salary> attribute.
Implementing Encapsulation with Classes
With the introduction of classes in ES6, encapsulation became more intuitive and aligned with other OOP languages:Understanding Inheritance in JavaScript
What is Inheritance?
Inheritance is another OOP principle that allows a class to inherit properties and methods from another class. This helps in code reusability and achieving a hierarchical relationship between classes.
Implementing Inheritance in JavaScript
Prior to ES6, inheritance was achieved through the prototype:With ES6 classes, inheritance became more straightforward using the ;extends> keyword:
By extending the ;Person> class, ;Employee> inherits its properties and methods, allowing for more complex and hierarchical object models.
Encapsulation and Inheritance: A Powerful Duo
When used together, encapsulation and inheritance offer a powerful way to organize and structure your JavaScript code. Encapsulation allows you to hide the internal state of an object and expose only what is necessary through a well-defined interface. Inheritance enables you to create a hierarchy of classes that share common functionality, reducing redundancy and enhancing the scalability of your code.
Understanding and applying these concepts are crucial steps toward becoming an adept JavaScript developer. As you continue to explore JavaScript, keep these principles in mind to craft efficient, maintainable, and robust web applications. Whether you’re working on simple scripts or complex web applications using WordPress or other frameworks, mastering encapsulation and inheritance will undoubtedly elevate your development skills.