Debugging Common Issues with Control Flow in JavaScript
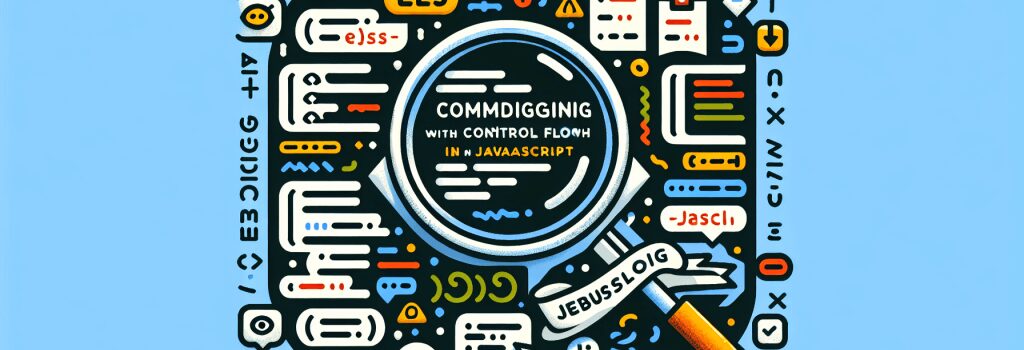
Introduction to Debugging JavaScript Control Flow
When embarking on the journey of web development, understanding the intricacies of JavaScript and its control flow mechanisms is paramount. Whether you’re writing simple scripts or complex applications, grasping how to debug common issues in JavaScript control flow can save you countless hours of frustration. This guide sheds light on identifying and fixing prevalent problems, making your coding process smoother and more efficient.
Understanding Control Flow in JavaScript
Control flow in JavaScript dictates the order in which the computer executes statements in a script. It’s the backbone of making decisions, looping through conditions, and executing functions based on those conditions. However, it can also be the source of various issues if not handled correctly.
Common Control Flow Statements
– if…else: Used for executing code blocks based on a condition.
– switch: Selects one of many code blocks to be executed.
– for: Executes a block of code a number of times with different values.
– while: Executes a block of code as long as a specified condition is true.
Debugging Common Control Flow Issues
Issue 1: Incorrect Use of Conditional Statements
One of the most common mistakes in JavaScript is the misuse of conditional statements like ;if…else>. Debugging this involves checking if the condition is correctly stated and ensuring it evaluates as expected.
Solution:
– Verify that the condition is correct and logically makes sense.– Use ;console.log()> to print out the condition’s value right before the ;if> statement to see what it evaluates to.
Issue 2: Infinite Loops in ;while> or ;for> Statements
Infinite loops occur when the loop’s exit condition is never met, potentially freezing your application.
Solution:
– Ensure your loop has a valid and reachable exit condition.– Debug by logging iteration counters or conditions within the loop to understand why the exit condition isn’t met.
Issue 3: Switch Statement Fall-through
A common issue with switch statements is forgetting to include a ;break>, causing unintended fall-through to other cases.
Solution:
– Double-check that each case concludes with a ;break> statement.– If intentional fall-through is desired, comment your code clearly to indicate this.
Issue 4: Misunderstanding Scope in Control Flows
JavaScript has function scope and block scope (with ;let> and ;const> in ES6). Misunderstandings here can lead to unexpected behaviors, especially within loops and conditional blocks.
Solution:
– Use ;let> and ;const> for block-scoped variables to avoid unintentional changes.– Understand the difference between ;var>, ;let>, and ;const> when declaring variables.
Issue 5: Asynchronous Code Execution
Asynchronous JavaScript, such as callbacks, promises, and async/await, can cause unexpected behavior when not properly handled within control flow structures.
Solution:
– Ensure asynchronous operations complete before their results are needed.– Use ;async/await> or promises to handle asynchronous operations more predictably within your control flow.
Best Practices for Debugging
– Use ;console.log()> Wisely: Log variables and conditions at various points in your control flow to understand the program’s state at those points.
– Utilize Browser Developer Tools: Modern browsers come equipped with powerful developer tools for debugging JavaScript. Familiarize yourself with breakpoints and the step-through feature.
– Comment and Refactor: Sometimes, rewriting a confusing block of code or adding comments for clarity can reveal issues you overlooked.
Conclusion
Debugging is an essential skill in web development. By understanding common issues with JavaScript control flow and employing strategic solutions, you can enhance your debugging efficiency. Remember, the key to successful debugging is patience and practice. With these strategies, you’ll be well-equipped to tackle control flow issues and develop more robust, error-free code.
Happy coding!