Creating and Manipulating Objects in JavaScript
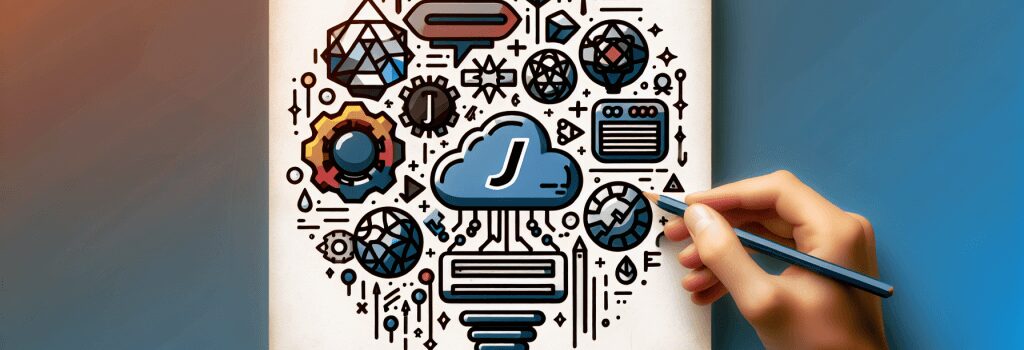
Creating and manipulating objects in JavaScript is a fundamental skill for any web developer looking to build dynamic and interactive web applications. Objects are the building blocks of JavaScript, enabling developers to store, manipulate, and manage data in an organized way. Through this article, you’ll gain insights into how to effectively create and manipulate objects, enhancing your web development skills.
Understanding Objects in JavaScript
Before diving into the creation and manipulation of objects, it’s crucial to understand what objects are in the context of JavaScript. An object is a collection of related data and functionality, which are stored as a series of key-value pairs. These keys can be strings or symbols, and the values can be anything from numbers, strings, arrays, to even other objects, allowing for a nested structure.
Object Creation in JavaScript
There are several ways to create objects in JavaScript, each with its own use cases and advantages.
Using Object Literals
The simplest way to create an object is by using the object literal syntax:
This method is straightforward and useful for creating single, unique objects.
Using the ;new Object()> Syntax
Another way to create an object is by using the ;new Object()> syntax:
This method provides a more dynamic approach to object creation, especially when creating multiple instances based on certain conditions.
Using Constructor Functions
Constructor functions allow for the creation of multiple instances of an object, facilitating code reusability and organization:
Manipulating Objects in JavaScript
Once objects are created, JavaScript provides numerous ways to manipulate them, including adding, modifying, and deleting properties.
Adding Properties
To add a new property to an object, simply assign a value to a new key on the object:
Modifying Properties
To modify an existing property, assign a new value to an existing key:
Deleting Properties
To remove a property, use the ;delete> operator:
Advanced Object Manipulation
JavaScript also offers advanced techniques for object manipulation, such as:
– Object.assign() to copy properties from one object to another.
– Object.keys() and Object.values() to retrieve an object’s keys and values, respectively.
– Object.freeze() to prevent modification to an object.
Conclusion
Understanding how to effectively create and manipulate objects in JavaScript is crucial for any aspiring web developer. By mastering these techniques, you’ll be able to build more structured, efficient, and powerful web applications. With practice, you’ll find that working with objects becomes a second nature, significantly enhancing your coding toolbox.