AJAX Requests in JavaScript: A Beginner’s Guide
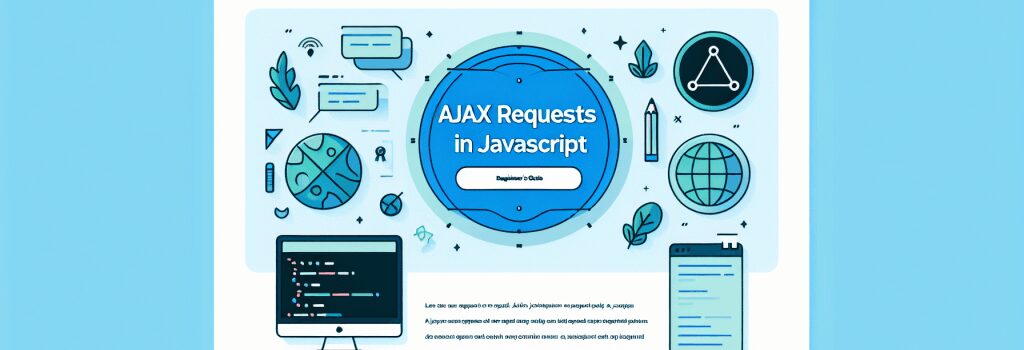
Understanding AJAX Requests in JavaScript
AJAX, which stands for Asynchronous JavaScript and XML, is a set of web development techniques that allows web applications to send and receive data asynchronously from a server without having to refresh the page. This technology is crucial for creating dynamic, fast, and interactive web applications. In this guide, we will explore the basics of making AJAX requests using JavaScript, an essential skill for aspiring web developers.
The Role of AJAX in Web Development
Before diving into the technicalities, it’s important to understand the importance of AJAX in modern web development. AJAX allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means that it is possible to update parts of a web page, without reloading the whole page, leading to a smoother, more engaging user experience.
How AJAX Works
AJAX requests are made using the ;XMLHttpRequest> object in JavaScript, which provides the capability to send and receive data asynchronously. With the advent of modern web development practices, the ;fetch> API has also become a popular, more modern way to make AJAX requests.
Making a Simple AJAX Request with the ;XMLHttpRequest> Object
1. Create an instance of ;XMLHttpRequest>
2. Establish a connection or initialize the request
3. Send the request
4. Handle the response
Using the Fetch API for AJAX Requests
The ;fetch> API provides a more powerful and flexible approach to making HTTP requests. It returns a Promise, making it easier to write cleaner code, especially when using async/await.
Handling Errors and Loading States
When making AJAX requests, it’s important to handle potential errors and loading states. This can be done by checking the status of the XMLHttpRequest or handling the catch block in a fetch request.
Conclusion
Understanding how to make AJAX requests is fundamental for any web developer looking to build dynamic and interactive web applications. While this guide covers the basics, practicing with different APIs and exploring further will deepen your understanding and proficiency in using AJAX in your projects. Whether you’re updating a web page without a full reload, submitting a form, or fetching data, mastering AJAX requests will significantly enhance the user experience of your web applications.