Utilizing JavaScript Promises for Efficient Async Operations
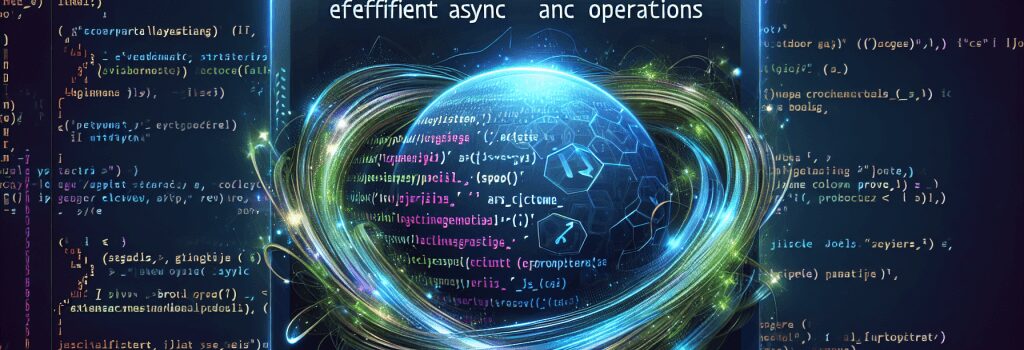
Let’s dive into a topic that’s essential for any web developer: JavaScript Promises. But wait now, dear reader, what is a Promise? No, it’s not when you swear you will stop making coding errors at 3 am. (Believe me, we’ve all been there.) A ‘Promise’ in JavaScript is an object that links the “producing code” and the “consuming code” together. This could, of course, lead to a long-term relationship, but let’s avoid getting mushy here.
In the real world, it would be ludicrous to assume that every operation is synchronous or happens one after the other. Just imagine doing all your chores one after the other, without multitasking. Saying you’d be “busy” would be a bit of an understatement. Now, in the world of web development, this is where JavaScript’s Promises come into play.
H2 Understanding Promises
Promises are a way to handle asynchronous operations in JavaScript. They are straightforward to use once you get a hang of them. Think of them as ordering at a drive-through. You’re in the back of a line but someone (or some drive-through operator) ‘promises’ to get your order to you. Now, this promise can go three ways:
1. Your order gets to you – the promise is fulfilled.
2. Your order completely messes up and you end up with a vegan burger when you asked for extra bacon – the promise is rejected.
3. Or they just stop responding entirely. What? Are you not fulfilling your promise, Corporate Sandwich Man?
In JavaScript land, a Promise object serves a similar purpose. It’s used for handling asynchronous operations, such as API requests.
H2 Creating Promises
To create a promise in JavaScript, you may use the Promise constructor. No, not the chap who builds houses, but the special function that creates an object.
This constructor takes in a function (known as the executor function) as a parameter that runs automatically when the Promise is created. This function, in turn, has two parameters, resolve and reject, which are functions used to indicate the completion or failure of the Promise.
Here is a small example:
const javaScriptPromise = new Promise((resolve, reject) => {
//your code here
});
JavaScript Promises are remarkably like that fitness plan you swear you’ll stick to this New Year. It has three states:
1. Pending: This is the default state of a Promise. This is like you, on the first of January – you neither have 6-pack abs yet, nor have you given up and raided your secret chocolate stash.
2. Fulfilled: This is when the Promise’s operation completes successfully, i.e., you bang out those pull-ups and crunches like they are nothing!
3. Rejected: This is when the Promise’s operation fails, i.e., you barely managed 2 pull-ups and went crying back to your aforementioned secret chocolate stash. No judgment here, pal.
H3 Handling Promises
Just like you catch your breath after a failed fitness attempt, in JavaScript, it’s crucial to handle both fulfilled and rejected Promises using the then() and catch() methods respectively.
Promise.then() method is used when a Promise is fulfilled, and Promise.catch() method is used when a Promise is rejected. Just keep remembering the fitness analogy, and you’re golden!
The world of JavaScript Promises can seem quite daunting at first. Don’t worry, it’s all part of the JavaScript rite of passage. Once you’ve tackled promises, you’ll be on your way to joining the ranks of advanced developers. Don’t forget, practice makes perfect, and the most effective way to understanding promises is by writing them. Happy coding!