Understanding PHP Arrays: A Beginner’s Guide
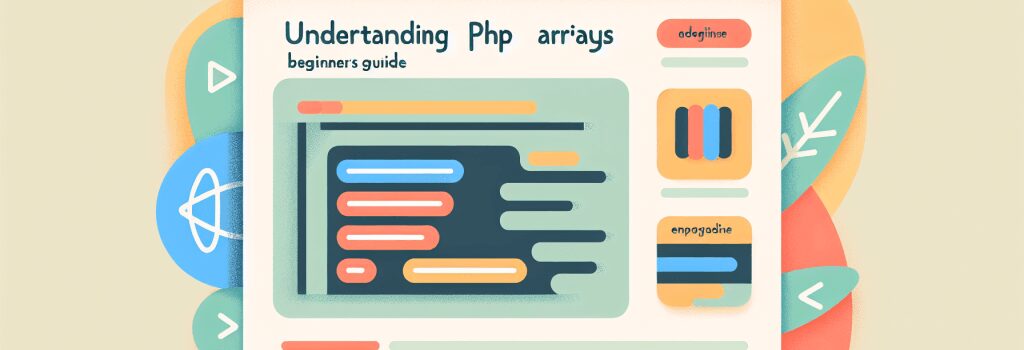
Introduction to PHP Arrays
PHP is a powerful scripting language that plays a pivotal role in web development, especially on the server side. Among its numerous capabilities, handling data structures such as arrays is a fundamental aspect that beginners must grasp. Arrays in PHP are versatile and can store multiple values within a single variable, streamlining data management and manipulation in your applications.
What are PHP Arrays?
PHP arrays are data structures that allow you to store multiple elements under a single name. Each element in the array can be accessed using its unique index or key. PHP supports three types of arrays:
– Indexed arrays – Arrays with a numeric index where values are stored linearly.
– Associative arrays – Arrays that use named keys that you assign to them.
– Multidimensional arrays – Arrays containing one or more arrays within them.
Understanding how to work with these arrays is crucial for backend development, data handling, and creating dynamic web pages.
Creating and Accessing PHP Arrays
Creating Indexed Arrays
Indexed arrays are the simplest form of arrays where the index is automatically assigned, starting from 0.
Creating Associative Arrays
Associative arrays allow you to associate a key to each value.
Accessing Array Elements
Access an element by specifying its index or key.
Manipulating PHP Arrays
PHP provides a wide range of functions to manipulate arrays.
Adding Elements
Removing Elements
Sorting Arrays
PHP has several functions for sorting arrays based on keys or values, in ascending or descending order.
Multidimensional Arrays in PHP
A multidimensional array contains one or more arrays. These are particularly useful for representing complex data structures.
Conclusion
Arrays are indispensable in PHP programming, facilitating the handling of grouped data with efficiency and simplicity. By mastering PHP arrays, you will be well-equipped to tackle diverse backend development challenges, manipulate data effectively, and contribute to building dynamic web applications. As you progress, experiment with the various array functions PHP offers, and explore how they can help streamline your data management tasks.
Understanding and implementing PHP arrays is a stepping stone into the vast world of web development. Embrace the journey, and you’ll find arrays to be powerful allies in your coding endeavors.