Serialization and Unserialization in PHP: Techniques and Tips
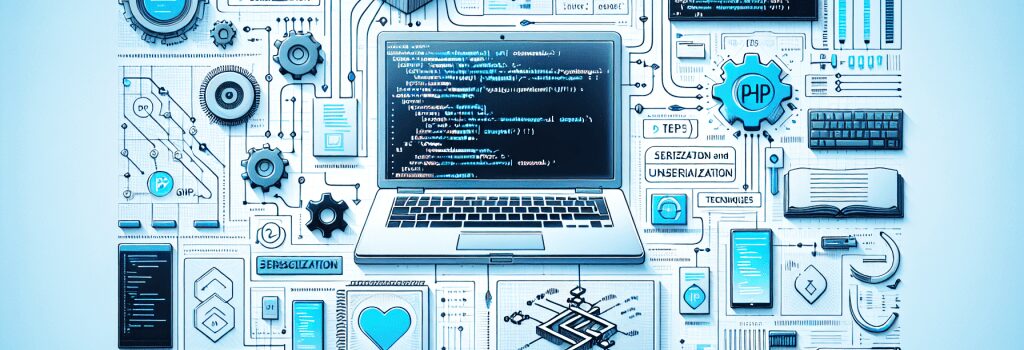
Hello there, budding web developers and eternal seekers of code wisdom. Get super comfortable, grab a bite of your favorite coding snack because we’re about to jump head-first into the world of PHP. Today, we’ll be diving deep into the mystical sphere of "Serialization and Unserialization in PHP: Techniques, and Tips". So, buckle up, remove your swim fins, because swimming isn’t much fun in the PHP ocean; it’s all about diving deep.
What is Serialization?
What is Serialization?
Let’s start at ground zero. Serialization? Sounds like something straight out of a sci-fi movie, right, sorts of saving humanity’s DNA for colonization on Mars. But fear not, there’s no weird science-gone-wrong here. Simply put, serialization in PHP is the act of converting a delicious, multi-layered PHP value (like an array or object) into a storable or transmitable string format. Makes space travel seem like a cakewalk, eh?Why use Serialization in PHP?
Why use Serialization in PHP?
Waving goodbye to our Mars colonizing dreams, let’s focus on why PHP’s serialization is actually useful. It’s like flattening a wonderfully crafted origami masterpiece, down to a piece of paper, then recreating the masterpiece at some different place (or time, if you still want to feel like a time lord). It helps to store or transmit data, perfect for use in cookies, sessions, databases or even HTTP/HTTPS requests.Equip Yourself with PHP Serialization
Equip Yourself with PHP Serialization
A word of caution: Buckle up, we’re ready for launch! PHP’s secret weapon for serialization: The serialize() function. With the precision of a master craftsman, it will convert your complex data into a compact string version without breaking a sweat!
<pre><code>php
$myArray = array("My", "first", "serialized", "array");
echo serialize($myArray);
Boom! You’ve just serialized your first array. High five!
Unserialize: Reversing the Spell
Unserialize: Reversing the Spell
After you’re done swooning over PHP’s serialization prowess, let’s master the art of reversing the spell. After all, what good is an origami masterpiece if it’s stuck being flat?Enter the unserialize() function. As the name suggests, it reverses the serialization process and reconstructs the original PHP value. It’s like watching your favorite summer blockbuster, but in reverse.
<pre><code>php
$str = 'a:4:{i:0;s:2:"My";i:1;s:5:"first";i:2;s:10:"serialized";i:3;s:5:"array";}';
print_r(unserialize($str));
And just like that, you’re no longer a one-trick pony!
A Few Quick Tips Before We Part
A Few Quick Tips Before We Part
While PHP’s serialization functions do all the nitty-gritty for you, there are a few points you need to be mindful of. Remember, with great power comes great responsibility or what I like to say during my code classes, "With great PHP knowledge, comes great need to debug".1. The serialized string by PHP is always Ascii, so no worries about special characters or Unicode.
2. If you’re trying to unserialize() something that isn’t properly formatted or was serialized by another language, PHP will return false, and might look pretty grumpy while at it.
3. Always be cautious when unserializing data passed from user-input. It’s like accepting a ticking package from the Coyote in a Road Runner episode.
And there you have it – all you need to navigate through the complexities of serialization and unserialization in PHP. Happy flipping through dimensions and keep having fun with PHP. See you in the next chapter, where we’ll uncover more of PHP’s wonders. Stay tuned and keep coding!
FAQ
What is serialization in PHP?
Serialization in PHP is the process of converting data structures or objects into a format that can be stored or transmitted. This format can be a string representation that can be reconstructed later.
What is unserialization in PHP?
Unserialization is the reverse process of serialization. It involves reconstructing a serialized string back into the original data structure or object.
Why is serialization important in PHP?
Serialization is important in PHP because it allows data to be easily stored, retrieved, and transferred between different systems or applications.
How can I serialize data in PHP?
You can serialize data in PHP using the `serialize()` function. This function takes a PHP value (array, object, etc.) and converts it into a storable representation.
Can all PHP data types be serialized?
Most PHP data types can be serialized, including arrays, objects, strings, integers, floats, and booleans. However, there are some data types like resources that cannot be serialized.
How can I unserialize data in PHP?
You can unserialize data in PHP using the `unserialize()` function. This function takes a serialized string and converts it back into the original PHP value.
Are there any risks associated with unserialization in PHP?
Yes, there are security risks associated with unserialization in PHP, especially when unserializing data from untrusted sources. Unserialization can potentially lead to code injection attacks.
How can I secure my application when using serialization and unserialization in PHP?
To secure your application when using serialization and unserialization, you should validate and sanitize input data, avoid unserializing data from untrusted sources, and consider using secure alternatives when possible.
When should I use serialization in PHP?
You can use serialization in PHP when you need to store or transfer complex data structures or objects, such as saving session data, caching data for later use, or passing data between different layers of an application.
Can I use serialization with databases in PHP?
While you can serialize data and store it in databases in PHP, it is generally not recommended for storing structured data. It can make querying and updating the data more complex. serialize data when saving it to the database.