Secure Your PHP Web Applications: Best Practices for Security
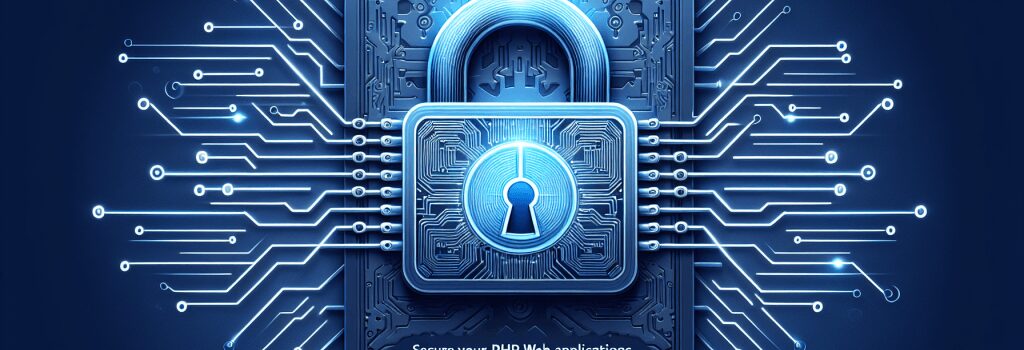
Introduction to PHP Security
In the realm of web development, securing your web applications is paramount. PHP, being a server-side scripting language used in backend development, powers a significant portion of the web. However, with great power comes great responsibility, especially in terms of security. Understanding and implementing security best practices can help safeguard your PHP applications against potential threats. This guide outlines essential security measures to enhance the protection of your PHP web applications.
Validate and Sanitize User Input
Importance of Input Validation
One of the fundamental principles of web security is to never trust user input. Malicious users can exploit vulnerabilities by injecting SQL, XSS, or other code into your applications through forms. Validating and sanitizing user input helps prevent these attacks.
Techniques for Input Validation
– Validate input formats: Use regular expressions or built-in PHP functions to ensure the data matches expected patterns.
– Sanitize data: Utilize PHP functions like ;filter_var()> with filters like ;FILTER_SANITIZE_STRING> to clean input data.
– Use prepared statements for database queries: This approach separates SQL logic from data, thwarting SQL injection attacks.
Manage Passwords Securely
Storing Passwords
Storing user passwords securely is crucial. Plain text or inadequately hashed passwords can be easily compromised.
Best Practices for Password Management
– Use PHP’s ;password_hash()> and ;password_verify()> functions: These functions provide robust hashing mechanisms, making it difficult for attackers to retrieve original passwords.
– Implement password policies: Encourage users to create strong, unique passwords and consider implementing multi-factor authentication for enhanced security.
Protect Against Cross-Site Scripting (XSS)
Understanding XSS Attacks
Cross-site scripting (XSS) attacks involve injecting malicious scripts into web pages viewed by other users. These attacks can lead to unauthorized access to user sessions, personal information, and more.
Mitigating XSS Risks
– Encode output: Escape HTML entities using functions like ;htmlspecialchars()> when displaying user input on web pages.
– Use Content Security Policy (CSP): Implementing CSP can significantly reduce the risks of XSS attacks by specifying which dynamic resources are allowed to load.
Implement Secure Session Management
Session Security Essentials
Compromised session IDs can lead to hijacking of user sessions. Protecting these IDs is hence a key aspect of web application security.
Strategies for Secure Sessions
– Use secure cookies: Set the ‘secure’ and ‘httpOnly’ flags on cookies to prevent access through client-side scripts.
– Regenerate session IDs: Especially after login, to prevent session fixation attacks.
– Timeout inactive sessions: Automatically log users out after a period of inactivity.
Conclusion
Securing PHP web applications is an ongoing process, requiring developers to stay vigilant and up-to-date with the latest security practices and threats. By adhering to these best practices—validating and sanitizing user input, managing passwords securely, protecting against XSS, and implementing secure session management—you can significantly enhance the security of your PHP web applications. Remember, investing in security is imperative to building trust and maintaining the integrity of your applications and users’ data.