PHP Regular Expressions for Efficient String Matching
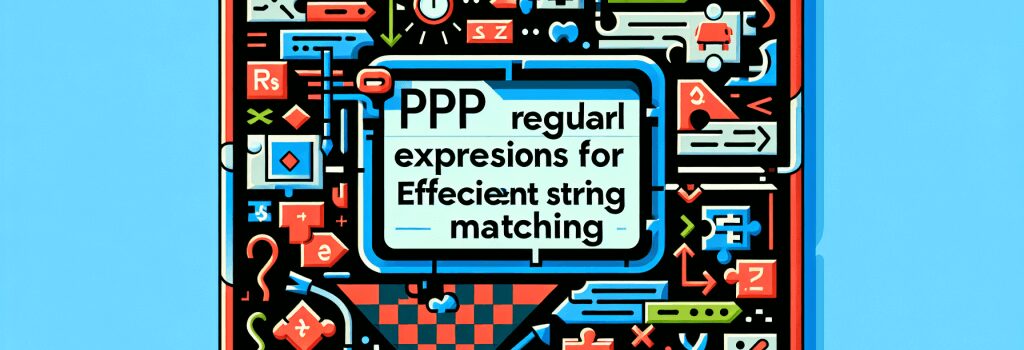
Alright beginner coders, welcome to one of the most thrilling – and at times, utterly hair-pulling – parts of being a back-end developer. Today, we’re going to dive deep into the magical world of PHP Regular Expressions.
Remember that time you were playing hide and seek, struggling to find that one mischievous friend? Well, Regular Expressions (regex) in PHP is sort of like that, but instead, you’re seeking matched patterns in strings. And trust me, those patterns can be very mischievous indeed.
So, buckle up, put on your detective glasses, and let’s get started!
What are Regular Expressions?
Before we jump into the specifics of how PHP regex works, let’s just shed some light on what regular expressions are. In short, regular expressions are a sequence of characters that form a search pattern. Now, don’t panic, I know the term ‘search pattern’ may sound like you’re about to do some detective work in Sherlock Holmes style, but in reality, it’s much simpler (although not quite as flashy).
PHP Regular Expressions – The Basics
Remember the super cool hacker movies where the protagonist is just typing some green text on a black screen? That’s not regular expressions. Sorry to burst the bubble, but remember, we’re dealing with real-life coding here, not Hollywood.
Now, PHP supports two styles of Regex – POSIX and Perl. Here, we’re not going to pick favorites, but we’re going to work with Perl because it’s the most commonly used, and frankly, the friendlier of the two.
In PHP, to use Perl style regex, you’ll make use of the preg_match() function. Now, put on your most comfortable coding hat and get cozy with the following syntax:
preg_match(‘pattern’, $subject)
Here ‘pattern’ is the regular expression you wish to match, and $subject is the string where you want to find the pattern. Simple, isn’t it?
The Mystery of the Patterns
Patterns in PHP regex start and end with a delimiter. The most commonly used delimiters are slashes (/), but you can also use hash (#) or tilde (~) among others. If you want to really feel like a Hollywood hacker, go wild and use different delimiters. I’ll stick with the good old slashes, thank you very much.
Strings can be a tricky business, just like that math equation you never quite understood back in seventh grade. But unlike those math classes, PHP’s regular expressions are on your side.
A Practical Look into PHP Regex
Let’s say you’re working with customer feedback and want to find all instances where customers mentioned ‘fantastic service’. Now, you can go through each feedback one by one, or you can be smart and use PHP regex. Here’s what using preg_match() would look like:
preg_match(‘/fantastic service/’, $feedback)
This will look for ‘fantastic service’ in your $feedback string and return a count of how many times they occur. If it doesn’t find anything, it just gives you an empty string. No drama, I promise!
Wrapping Up
I know, PHP regex can seem scary at first, like facing a lion on your first day as a zookeeper. But it’s actually a very useful tool, especially when it comes to working with strings in PHP.
Remember, dear reader, we ain’t coding kittens, we’re coding lions! Take some time to play around with different regular expressions and get familiar with the syntax. Before you know it, you’ll be detecting patterns like a pro, and Regex won’t seem as fearsome as before.
Until next time – happy coding!