PHP Error Handling with Try-Catch Blocks for Robust Applications
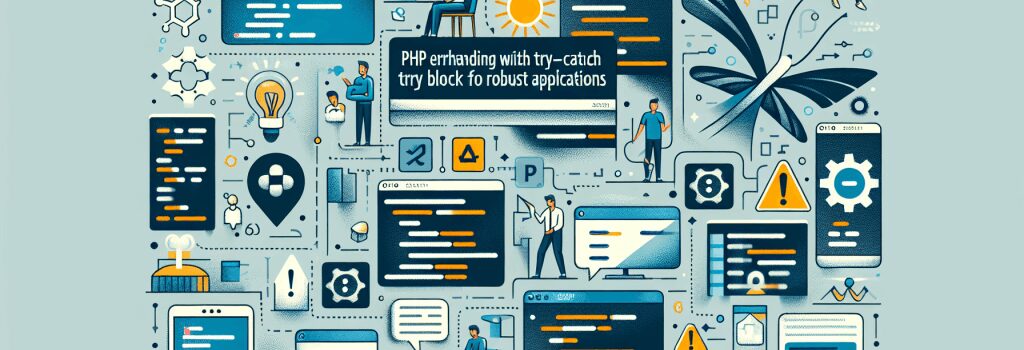
—PHP is a powerful server-side scripting language widely used in web development. While crafting PHP applications, error handling becomes crucial to ensure the robustness and reliability of your applications. Employing try-catch blocks in PHP can significantly enhance your error management strategy.
Understanding Try-Catch in PHP
Try-catch blocks in PHP are a part of exception handling mechanism. An exception is an object that describes an error or unexpected behavior encountered during the execution of a script. When an exception is thrown, the normal flow of execution halts, and PHP searches for a matching "catch" block to handle the exception.
Syntax of Try-Catch in PHP:
Benefits of Using Try-Catch for Error Handling
– Improved Error Management: By encapsulating potential error-generating code within a try block, you can elegantly manage errors with corresponding catch blocks.
– Code Maintainability: Segregating error handling code from the main logic makes your code more readable and maintainable.
– Custom Error Messages: Catch blocks allow you to display custom error messages or perform specific actions based on different types of exceptions.
Implementing Try-Catch in PHP
Basic Try-Catch Block
Consider a situation where you’re trying to connect to a database. Database connection issues are common, and handling them properly is crucial for the user experience.
In this example, if the PDO class fails to connect to the database, it throws a ;PDOException>. The catch block catches this exception and displays a user-friendly message.
Catching Multiple Exceptions
PHP 7 introduced the capability to catch multiple exception types in a single catch block, enhancing error handling flexibility.
This feature is particularly useful when different operations might throw different exceptions, and you want to handle all of them in a similar manner.
Best Practices for Error Handling in PHP
– Throwing Exceptions: Consider throwing exceptions in your code to signal that an error has occurred. This makes your code more modular and easier to debug.
– Custom Exceptions: Define custom exception classes for more granular error handling. This practice allows you to catch specific exceptions and handle them accordingly.
– Finally Block: Use the ;finally> block if you have code that must be executed regardless of whether an exception is caught, such as closing a file or releasing resources.
– Logging: Always log your exceptions, as this information is invaluable for debugging purposes.
Conclusion
Effective error handling is a hallmark of robust applications. By leveraging try-catch blocks in PHP, developers can write more reliable, maintainable, and user-friendly web applications. Remember, the goal is not only to prevent errors but also to handle them gracefully when they occur.
Incorporating these practices into your PHP development workflow will significantly enhance the quality of your applications, making them more resilient against unexpected issues and improving the overall user experience.
—By mastering the concepts of exception handling and error management in PHP, you’re taking a significant step towards becoming a proficient web developer. Remember, robust applications are not just about flawless code but about how they stand up to and recover from errors.