PHP Array Functions: Sorting, Searching, and More
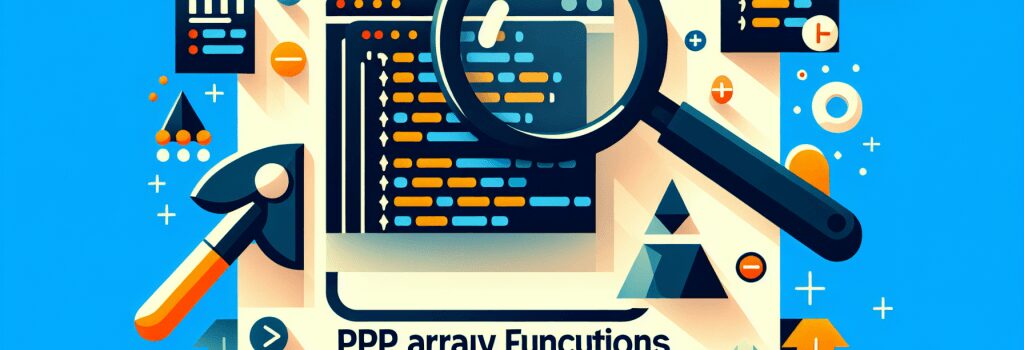
Understanding PHP Array Functions: A Complete Guide
Arrays are a fundamental structure in PHP, offering a flexible way to store and manipulate data. With arrays, you can collect, process, and output data efficiently. PHP provides a wide range of built-in functions to work with arrays, from sorting and searching to more advanced operations. This article dives deep into some of the most useful PHP array functions, helping you to leverage their power in your web development projects.
Sorting Arrays in PHP
Sorting is a recurring requirement in web development, whether you’re ordering posts by date, products by price, or users by their names. PHP offers various functions to sort arrays depending on your needs.
sort() and rsort()
The ;sort()> function is used to sort arrays in ascending order, while ;rsort()> sorts arrays in descending order. These are useful for numeric or string arrays where the elements need to be arranged either from low to high or vice versa.
asort() and arsort()
When dealing with associative arrays, where maintaining the association between keys and values is crucial, ;asort()> and ;arsort()> come in handy. ;asort()> sorts the array in ascending order according to the value while keeping the key association intact, and ;arsort()> does the same in descending order.
usort(), uasort(), and uksort()
PHP also allows custom sorting through user-defined functions. ;usort()> sorts an array by values using a comparison function you define, ;uasort()> does the same while preserving keys, and ;uksort()> sorts the array by keys.
Searching Arrays in PHP
Searching is another key operation with arrays, allowing developers to find if an element exists, retrieve its value, or fetch its key.
in_array()
The ;in_array()> function checks if a given value exists in an array, returning ;true> if it does and ;false> otherwise. This is particularly useful for conditional checks.
array_search()
To find the key of a matching value, ;array_search()> is the go-to function. If the value is found in the array, the key is returned. Otherwise, it returns ;false>.
array_keys() and array_values()
Sometimes, getting all keys or values from an array is necessary. ;array_keys()> returns an array of all the keys, and ;array_values()> fetches all the values.
More Useful PHP Array Functions
Beyond sorting and searching, PHP arrays come with a lot more versatility through various functions.
array_merge()
Combining arrays is a common task, and ;array_merge()> seamlessly merges the elements of one or more arrays together. This is particularly useful when working with data from different sources.
array_diff() and array_intersect()
Comparing arrays can help identify differences or similarities. ;array_diff()> returns the values from the first array that are not present in the other arrays. On the other hand, ;array_intersect()> finds all the values that are present in all the arrays.
count()
Knowing the size of an array is crucial for loops and conditional statements. The ;count()> function gives you the number of elements in an array.
Conclusion
PHP’s array functions provide developers with a powerful toolkit for data manipulation, enabling more efficient and effective coding practices. By understanding how to sort, search, and manipulate arrays in PHP, you can significantly enhance your web development projects. Remember, the key to mastering PHP arrays lies in practice and experimentation, so don’t hesitate to try out these functions in your next project.