Logical Operators in PHP: Making Decisions in Your Code
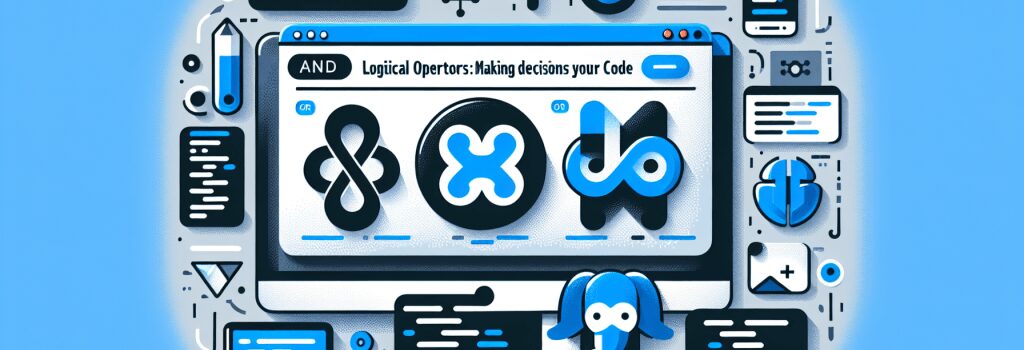
Logical operators play a crucial role in any programming language, especially in PHP, where making conditional decisions is a daily task for developers. These operators allow programs to execute code based on multiple conditions, making your applications dynamic and intelligent. Understanding how to utilize logical operators in PHP will significantly enhance your web development skills. Here’s a comprehensive guide to mastering logical operators in PHP.
Understanding Logical Operators in PHP
At its core, PHP facilitates decision-making in your code through conditional statements. Logical operators are the backbone of these statements, allowing you to combine multiple conditions and make decisions based on the result. Logical operators evaluate to either ;true> or ;false>, making them essential for controlling the flow of your applications.
The Basic Logical Operators in PHP
PHP supports several logical operators, each serving a unique purpose:
– AND (&& or and): This operator returns ;true> if both operands are true. It’s perfect for combining conditions that must all be met.
– OR (|| or or): Returns ;true> if at least one of the operands is true. Use this when you have multiple possible conditions for the same outcome.
– XOR: A less common operator, XOR (exclusive or) returns ;true> if either operand is true, but not both. It’s useful for toggling states.
– NOT (!): This operator inverts the truth value of its operand. If a condition is ;true>, NOT will make it ;false>, and vice versa. It’s ideal for reversing conditions.
Practical Examples of Logical Operators in Action
Let’s put these operators into perspective with some practical PHP code examples.
<h4>Using the AND OperatorIn this example, access is only granted if both conditions are ;true>: the user is logged in and has the necessary permissions.
<h4>Exploring the OR OperatorHere, a customer receives a discount if they have a discount code or are shopping for the first time.
<h4>The Power of the NOT OperatorThe NOT operator is used to invert the ;$is_admin> condition. The message is displayed only if ;$is_admin> is ;false>.
Combining Logical Operators
Logical operators can be combined to form complex conditions. However, it’s crucial to use parentheses for clarity and to ensure the correct evaluation order.
In this scenario, access to premium content is granted if the user is logged in with premium access or has a discount code.
Logical Operators: Best Practices
When using logical operators:
– Readability is key: Always aim for clear and understandable code. If an expression becomes too complex, consider breaking it down into smaller parts.
– Use parentheses: This enhances readability and ensures conditions are evaluated as intended.
– Understand operator precedence: Knowing the order in which PHP evaluates different operators can prevent unexpected results.
Mastering logical operators in PHP is essential for making decisions in your code, thereby making your web applications dynamic and responsive. By understanding and utilizing these operators effectively, you can control the flow of execution in your applications, leading to more robust and reliable code. Happy coding!