Creating Your First PHP Web Application: A Step-by-Step Guide
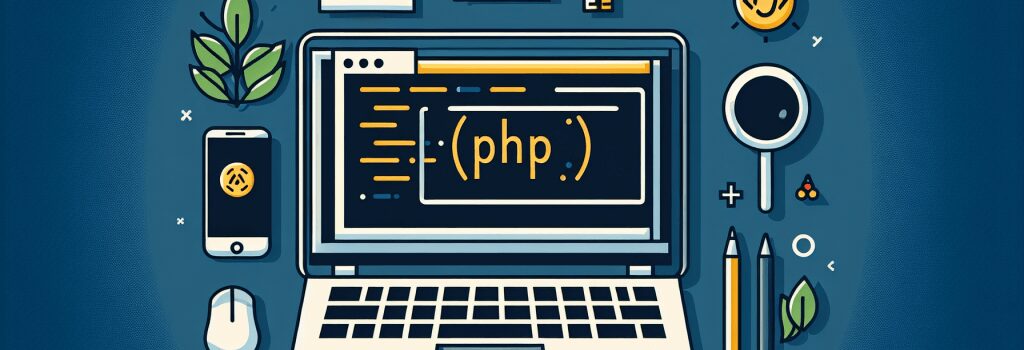
Embarking on the journey to create your first web application with PHP and MySQL is an exciting venture into the world of backend development. PHP, a server-side scripting language, combined with MySQL, a powerful database management system, provides a robust foundation for building dynamic and interactive web applications. This step-by-step guide will walk you through the process of setting up your development environment, writing your first PHP script, connecting to a MySQL database, and finally, creating a simple web application. By following this guide, you’ll gain the foundational skills needed to dive deeper into the realm of web development.
Setting Up Your Development Environment
Before you start coding, it’s essential to have a development environment ready. This involves installing a software stack that includes PHP, MySQL, and a web server like Apache or Nginx. Solutions like XAMPP or MAMP can simplify this process by bundling these components together, making installation and configuration straightforward for beginners.
Installing XAMPP or MAMP
1. Download the XAMPP or MAMP installer package from their respective websites.
2. Follow the installation instructions specific to your operating system.
3. Once installed, start the servers using their control panel.
Ensure that both Apache (or Nginx) and MySQL are running before proceeding.
Writing Your First PHP Script
With your development environment set up, it’s time to write your first PHP script. PHP scripts are executed on the server and can generate dynamic content before sending it to the client’s browser.
Creating a Simple Script
1. Open your text editor or IDE and create a new file named ;hello.php>.
2. Type the following PHP code:
3. Save the file in the ;htdocs> (XAMPP) or ;www> (MAMP) directory.
4. Access the script by navigating to ;http://localhost/hello.php> in your web browser. You should see "Hello, world!" displayed on the page.
Connecting to a MySQL Database
Now that you’ve successfully written a PHP script, the next step is to connect it to a MySQL database. This is crucial for creating dynamic applications that require data storage and retrieval.
Creating a Database and Table
1. Open the PHPMyAdmin from your XAMPP or MAMP control panel.
2. Create a new database named ;test_db>.
3. Within ;test_db>, create a table named ;greetings> with two columns: ;id> (INT, primary key, auto_increment) and ;message> (VARCHAR).
Connecting PHP to MySQL
1. Create a new PHP file named ;dbconnect.php>.
2. Use the ;mysqli_connect()> function to connect to your database:
Replace ;"yourUsername"> and ;"yourPassword"> with your MySQL credentials.
Creating a Simple Web Application
Now that you have your development environment ready, a basic understanding of PHP scripts, and a connection to a MySQL database, it’s time to create a simple web application. This application will display a greeting message stored in the database.
1. Insert a greeting message into the ;greetings> table using PHPMyAdmin.
2. Modify ;hello.php> to fetch the greeting message from the database and display it:
Wrapping Up
Congratulations! You’ve just created your first PHP web application. This guide introduced you to PHP and MySQL, taught you how to set up a development environment, write PHP scripts, interact with a MySQL database, and ultimately build a simple web application.
Remember, this is just the beginning. As you explore more about PHP and MySQL, you’ll discover a wide range of functionalities and best practices that will enhance your ability to create more complex and robust web applications. Happy coding!