Creating Dynamic Web Forms with PHP: A Step-by-Step Guide.
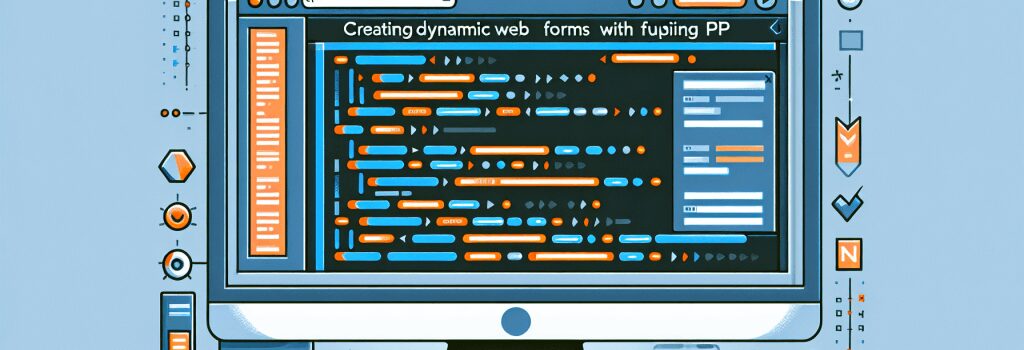
Introduction to Dynamic Web Forms with PHP
Dynamic web forms are at the heart of interactive websites, enabling users to submit data that web applications can process in real-time. PHP, with its ease of use and integration with HTML, CSS, and JavaScript, stands out as a powerful tool for creating such forms. This guide will walk you through the process of constructing dynamic web forms with PHP, from handling simple inputs to validating and storing the submitted data.
Understanding the Basics of PHP Forms
Form Creation with HTML
Before diving into PHP, you must understand how to create a form with HTML. A basic form includes various elements like input fields, labels, and a submit button. Here’s an example:
The ;action> attribute points to the PHP file that will process the form data, and the ;method> attribute specifies how the data should be sent (;post> or ;get>).
Processing Form Data with PHP
Once the form is submitted, the processing file (;submit.php> in our example) will handle the data. Accessing the data is straightforward with PHP’s ;$_POST> or ;$_GET> arrays, depending on your form’s method. For a form submitted via POST, you can retrieve the user’s name as follows:
Handling Data Validation and Security
Data Validation with PHP
Validating form data is crucial to prevent errors and ensure the data matches expected formats. PHP offers functions like ;filter_var()> for basic data validation. For instance, validating an email address could look like this:
Security Considerations
When dealing with form data, security should never be overlooked. Always sanitize user inputs to avoid SQL injections and cross-site scripting (XSS) attacks. PHP provides several functions for sanitization, such as ;htmlspecialchars()> and ;filter_var()> with the ;FILTER_SANITIZE_STRING> filter.
Storing Form Data
Connecting to a Database
To store form data, you’ll likely interact with a database. PHP’s PDO (PHP Data Objects) extension offers a secure and flexible way to connect to a database. Here’s a basic PDO connection setup to a MySQL database:
Inserting Form Data into a Database
After establishing a connection, you can insert the data using prepared statements, which also prevent SQL injection:
Conclusion
Creating dynamic web forms with PHP is a fundamental skill for web developers. By understanding form creation, data validation, and secure data handling, you’re well on your way to enhancing the interactivity and functionality of your web applications. Remember, practice is key to mastering PHP form handling, so don’t hesitate to experiment with more complex forms and validation rules.
Incorporating PHP into your web development stack offers a robust solution for creating dynamic, user-friendly websites. As you continue to explore PHP’s capabilities, you’ll discover even more ways to leverage its power in backend development.