Creating and Using Functions in PHP for Efficient Coding
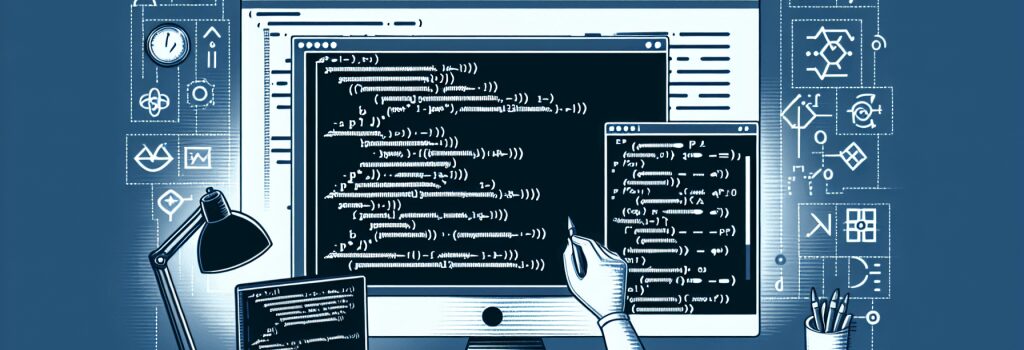
Introduction to Functions in PHP
Programming in PHP is both a craft and an art. One of the fundamental skills that set the foundation for efficient coding in PHP is mastering functions. A function is essentially a block of code designed to perform a particular task. When you find yourself repeating code in your applications, it’s time to think about wrapping that code in a function. This allows for better code reusability, easier maintenance, and more readable code. In this comprehensive guide, we’ll delve into creating and using functions in PHP to elevate your coding efficiency and readability.
Understanding PHP Functions
PHP offers a vast library of built-in functions that can perform a wide range of tasks. However, the power of PHP functions really shines through when you start to create your own. A custom function in PHP is declared with the ;function> keyword, followed by a unique function name and a pair of parentheses ;()> which can optionally contain parameters.
Syntax of PHP Functions
The basic syntax for declaring a function in PHP is as follows:
Parameters are variables that act as placeholders for the values that you will pass to the function when you call it. They are optional; a function may not require any parameters.
Creating Your First PHP Function
Let’s dive into an example. Suppose you frequently need to greet users on a website. Instead of writing the greeting code each time, you can encapsulate it within a function:
To use the function, you call it by its name followed by passing the required parameters within parentheses:
Parameters and Return Values
Functions can return values back to the part of the program where they were called. To do this, use the ;return> statement. The ;return> statement immediately ends the function’s execution and sends back the specified value.
Consider a function that adds two numbers:
Advanced Concepts
Default Parameter Values
You can set default values for the function parameters. This means if no value is passed to the function, it uses the default.
Passing Arguments by Reference
Normally, PHP passes arguments to functions by value. However, you can pass them by reference using the ;&> sign. This means any changes to the parameter inside the function will affect the original variable.
Conclusion
Mastering functions in PHP is crucial for writing efficient, readable, and maintainable code. By encapsulating code in functions, you can avoid redundancy, making your applications cleaner and more organized. Start experimenting with creating your own functions, keeping in mind the ability to use parameters and return values to make your functions more dynamic and versatile.
By understanding and applying these principles, you’re on your path to becoming an effective PHP developer. Remember, practice is key in programming. So, embrace functions in your next project and watch your coding capabilities grow exponentially.